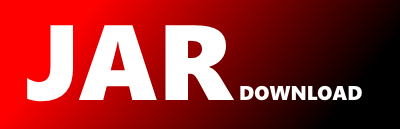
com.google.sitebricks.headless.Reply Maven / Gradle / Ivy
package com.google.sitebricks.headless;
import java.io.IOException;
import java.util.Map;
import javax.servlet.http.HttpServletResponse;
import com.google.inject.Injector;
import com.google.inject.Key;
import com.google.sitebricks.client.Transport;
/**
* Reply to a (headless) web request.
*/
public abstract class Reply {
// Asks sitebricks to continue down the servlet processing chain
public static final Reply> NO_REPLY = Reply.saying();
public static final String NO_REPLY_ATTR = "sb_no_reply";
/**
* Perform a 301 redirect (moved permanently) to the given uri.
*/
public abstract Reply seeOther(String uri);
/**
* Perform a custom redirect to the given uri. The status code must be
* in the 3XX range.
*/
public abstract Reply seeOther(String uri, int statusCode);
/**
* The media type of the response data to send to the client. I.e.
* the mime-type. Example {@code "application/json"} for JSON responses.
*/
public abstract Reply type(String mediaType);
/**
* A Map of headers to set directly on the response.
*/
public abstract Reply headers(Map headers);
/**
* Perform a 404 not found reply.
*/
public abstract Reply notFound();
/**
* Perform a 401 not authorized reply.
*/
public abstract Reply unauthorized();
/**
* Directs sitebricks to use the given Guice key as a transport to
* marshall the provided entity to the client. Example:
*
* return Reply.with(new Person(..)).as(Xml.class);
*
*
* Will marhall the given Person object into XML using the Guice Key
* bound to [Xml.class] (by default this is an XStream based XML
* transport).
*/
public abstract Reply as(Class extends Transport> transport);
/**
* Same as {@link #as(Class)}.
*/
public abstract Reply as(Key extends Transport> transport);
/**
* Perform a 302 redirect to the given uri (moved temporarily).
*/
public abstract Reply redirect(String uri);
/**
* Perform a 403 resource forbidden error response.
*/
public abstract Reply forbidden();
/**
* Perform a 204 no content response.
*/
public abstract Reply noContent();
/**
* Perform a 500 general error response.
*/
public abstract Reply error();
/**
* Render template associated with the given class. The class must have
* an @Show() annotation pointing to a valid Sitebricks template type (can
* be any of the supported templates: MVEL, freemarker, SB, etc.)
*
* The entity passed into with() is used as the template's context during
* render.
*/
public abstract Reply template(Class> templateKey);
/**
* Set a custom status code (call this last, it will be overridden if
* other response code directives are called afterward).
*/
public abstract Reply status(int code);
/**
* Perform a 200 OK response with no body.
*/
public abstract Reply ok();
/**
* Used internally by sitebricks. Do NOT call.
*/
abstract void populate(Injector injector, HttpServletResponse response) throws IOException;
/**
* Convenience method to make a reply without any entity or body. Example, to send a redirect:
*
* return Reply.saying().redirect("/other");
*
*/
public static Reply saying() {
return new ReplyMaker(null);
}
/**
* Returns a reply with an entity that is sent back to the client via the specified
* transport.
*
* @param entity An entity to send back for which a valid transport exists (see
* {@link #as(Class)}).
*/
public static Reply with(E entity) {
return new ReplyMaker(entity);
}
}