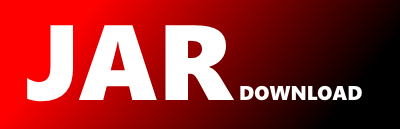
br.com.objectos.testable.IterableEquality Maven / Gradle / Ivy
/*
* Copyright 2015 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.testable;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Objects;
/**
* @author [email protected] (Marcio Endo)
*/
class IterableEquality implements Equality {
private final String name;
private final List resultList;
private IterableEquality(String name, List resultList) {
this.name = name;
this.resultList = resultList;
}
public static Equality of(String name, Iterable> a, Iterable> b) {
if (a == b) {
return Equality.equal();
}
List resultList = new ArrayList<>();
if (b == null) {
resultList.add(Equality.nullValue());
return new IterableEquality(name, resultList);
}
Iterator> iterA = a.iterator();
Iterator> iterB = b.iterator();
int index = -1;
while (iterA.hasNext()) {
index++;
Object nextA = iterA.next();
if (!iterB.hasNext()) {
resultList.add(Equality.elementMissing(index, nextA));
break;
}
Object nextB = iterB.next();
Equality equality = nextA instanceof Testable
? ((Testable) nextA).isEqualTo(nextB)
: Objects.equals(nextA, nextB)
? Equality.equal()
: Equality.elementNotEqual(index, nextA, nextB);
resultList.add(equality);
}
while (iterB.hasNext()) {
index++;
Object nextB = iterB.next();
resultList.add(Equality.elementNotExpected(index, nextB));
}
return new IterableEquality(name, resultList);
}
@Override
public boolean isEqual() {
boolean isEqual = true;
for (Equality equality : resultList) {
if (!equality.isEqual()) {
isEqual = false;
break;
}
}
return isEqual;
}
@Override
public void accept(Reporter reporter) {
reporter.name(name);
for (Equality equality : resultList) {
equality.accept(reporter);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy