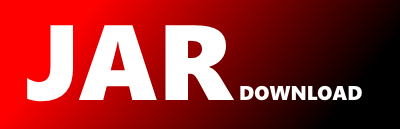
br.com.objectos.testable.Reporter Maven / Gradle / Ivy
/*
* Copyright 2015 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.testable;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.stream.Collectors;
/**
* @author [email protected] (Marcio Endo)
*/
public class Reporter {
private final List lineList = new ArrayList<>();
private int level;
private Reporter() {
}
public static Reporter start() {
return new Reporter();
}
public void elementMissing(int index, String expected) {
line("element[%d] is missing. Expected <%s>.", index, expected);
}
public void elementNotEqual(int index, String expected, String found) {
line("element[%d] not equal. Expected <%s> found <%s>", index, expected, found);
}
public void elementNotExpected(int index, String found) {
line("element[%d] not expected. Found <%s>", index, found);
}
public void fail(String expected, String found) {
line("expected: %s", expected);
line(" found: %s", found);
}
public void foundNull(String name) {
line("- %s: found null", name);
}
public void indent() {
level++;
}
public List lineList() {
return Collections.unmodifiableList(lineList);
}
public void name(String name) {
line("- %s", name);
}
public void notEqual(String name, String a, String b) {
name(name);
fail(a, b);
}
public void nullValue() {
line("- value is null");
}
public void test() throws AssertionError {
if (!lineList.isEmpty()) {
throwAssertionError();
}
}
@Override
public String toString() {
return lineList.stream().collect(Collectors.joining("\n"));
}
public void throwAssertionError() throws AssertionError {
throw new AssertionError(toString());
}
public void typeNotEqual(Class> typeA, Class> typeB) {
line("- types are not equal");
line("expected: %s", typeA);
line(" found: %s", typeB);
}
public void unindent() {
level--;
}
private void line(String format, Object... args) {
StringBuilder msg = new StringBuilder();
for (int i = 0; i < level * 2; i++) {
msg.append(' ');
}
msg.append(String.format(format, args));
lineList.add(msg.toString());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy