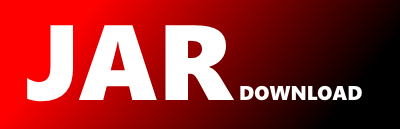
br.com.objectos.tftp.ReadRequestMessage Maven / Gradle / Ivy
/*
* Copyright 2016 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.tftp;
import java.nio.ByteBuffer;
import java.util.List;
import br.com.objectos.net.ByteBuffers;
import br.com.objectos.udp.Datagram;
import br.com.objectos.udp.DatagramSpec;
import br.com.objectos.udp.DatagramSpec.DatagramBuilder;
import br.com.objectos.udp.Field;
import br.com.objectos.udp.Message;
import br.com.objectos.udp.Packet;
/**
* @author [email protected] (Marcio Endo)
*/
public class ReadRequestMessage implements Message {
static final Field OPCODE = OpCode.RRQ.field();
static final Field FILENAME = TftpString.field("filename");
static final Field MODE = Mode.field();
static final Field OPTIONS = Options.field();
private static final DatagramSpec SPEC = DatagramSpec.builder()
.add(OPCODE)
.add(FILENAME)
.add(MODE)
.add(OPTIONS)
.build();
private final Datagram datagram;
private ReadRequestMessage(Datagram datagram) {
this.datagram = datagram;
}
static ReadRequestMessage get(String filename) {
return ReadRequestMessage.builder()
.put(FILENAME, TftpString.of(filename))
.put(MODE, Mode.OCTET)
.build();
}
static ReadRequestMessage read(ByteBuffer buffer) {
System.out.println(ByteBuffers.toHexString(buffer));
Datagram datagram = SPEC.read(buffer);
return new ReadRequestMessage(datagram);
}
private static DatagramBuilder builder() {
return SPEC.datagramBuilder(ReadRequestMessage::new);
}
@Override
public Datagram datagram() {
return datagram;
}
public void execute(Server server, Packet packet) {
String filename = datagram.get(FILENAME).toString();
byte[] bytes = server.open(filename);
TftpFile file = new TftpFile(bytes.length);
OAckMessage oackMessage = options().toSupported(file).toOAckMessage();
ReadOptions options = oackMessage.toReadOptions();
List messageList = options.toDataMessage(bytes);
ReadSession session = ReadSession.of(packet, oackMessage, messageList);
session.start(server);
}
public String filename() {
return datagram.get(FILENAME).toString();
}
public Mode mode() {
return datagram.get(MODE);
}
public TftpString option(OptionType type) {
return options().get(type);
}
@Override
public String toString() {
return datagram.toString();
}
private Options options() {
return datagram.get(OPTIONS);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy