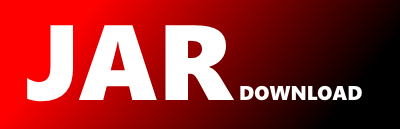
br.com.objectos.way.reports.htmltopdf.HtmltopdfGuice Maven / Gradle / Ivy
/*
* Copyright 2013 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.reports.htmltopdf;
import java.io.File;
import com.google.common.base.Preconditions;
import com.google.inject.Inject;
/**
* @author Marcio Endo ([email protected])
*/
class HtmltopdfGuice implements Htmltopdf {
private final HtmltopdfDelegate delegate;
@Inject
public HtmltopdfGuice(HtmltopdfDelegate delegate) {
this.delegate = delegate;
}
@Override
public Authenticate authenticateTo(String authUrl) {
return Config.forAuthenticate(delegate, authUrl);
}
@Override
public Get get(String url) {
return Config.forGet(delegate, url);
}
static class Config implements Authenticate, Get, HtmltopdfOptions {
private final HtmltopdfDelegate delegate;
private String url;
private File file;
private AuthInfo authInfo;
private final Page page = new Page();
private HeaderFooter header;
private HeaderFooter footer;
private Config(HtmltopdfDelegate delegate) {
this.delegate = delegate;
}
public static Config forAuthenticate(HtmltopdfDelegate delegate, String authUrl) {
return new Config(delegate).authUrl(authUrl);
}
public static Config forGet(HtmltopdfDelegate delegate, String url) {
return new Config(delegate).url(url);
}
public Config authUrl(String authUrl) {
this.authInfo = new AuthInfo(authUrl);
return this;
}
public Config url(String url) {
this.url = url;
return this;
}
@Override
public Authenticate post(String name, String value) {
authInfo.post(name, value);
return this;
}
@Override
public Get get(String url) {
this.url = url;
return this;
}
@Override
public Get toPaperFormat(PaperFormat format) {
PaperSize size = page.getPaperSize();
size.format(format);
return this;
}
@Override
public Get orientedAs(Orientation orientation) {
PaperSize size = page.getPaperSize();
size.orientation(orientation);
return this;
}
@Override
public WithMargins withMargins() {
PaperSize size = page.getPaperSize();
Margin margin = size.getMargin();
return new WithMarginsImpl(margin);
}
@Override
public Get withoutMargins() {
PaperSize size = page.getPaperSize();
size.withoutMargins();
return this;
}
@Override
public WithHeader withHeader() {
header = new HeaderFooter();
return new WithHeaderImpl(header);
}
@Override
public WithFooter withFooter() {
footer = new HeaderFooter();
return new WithFooterImpl(footer);
}
@Override
public File andWriteTo(File file) {
Preconditions.checkNotNull(url);
Preconditions.checkNotNull(file);
this.file = file;
delegate.render(this);
return file;
}
// HtmltopdfOptions
@Override
public String getUrl() {
return url;
}
@Override
public File getFile() {
return file;
}
@Override
public AuthInfo getAuthInfo() {
return authInfo;
}
@Override
public Page getPage() {
return page;
}
@Override
public HeaderFooter getHeader() {
return header;
}
@Override
public HeaderFooter getFooter() {
return footer;
}
@Override
public String toString() {
return url;
}
private class WithMarginsImpl implements WithMargins {
private final Margin margin;
public WithMarginsImpl(Margin margin) {
this.margin = margin;
}
@Override
public WithMargins top(int size, Unit unit) {
margin.top(size, unit);
return this;
}
@Override
public WithMargins right(int size, Unit unit) {
margin.right(size, unit);
return this;
}
@Override
public WithMargins bottom(int size, Unit unit) {
margin.bottom(size, unit);
return this;
}
@Override
public WithMargins left(int size, Unit unit) {
margin.left(size, unit);
return this;
}
@Override
public Get resized() {
return Config.this;
}
}
private class WithHeaderImpl implements WithHeader {
private final HeaderFooter header;
private WithHeaderImpl(HeaderFooter header) {
this.header = header;
}
@Override
public WithHeader height(int size, Unit unit) {
header.height(size, unit);
return this;
}
@Override
public WithHeader contents(String contents) {
header.contents(contents);
return this;
}
@Override
public Get customized() {
return Config.this;
}
}
private class WithFooterImpl implements WithFooter {
private final HeaderFooter footer;
private WithFooterImpl(HeaderFooter footer) {
this.footer = footer;
}
@Override
public WithFooter height(int size, Unit unit) {
footer.height(size, unit);
return this;
}
@Override
public WithFooter contents(String contents) {
footer.contents(contents);
return this;
}
@Override
public Get customized() {
return Config.this;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy