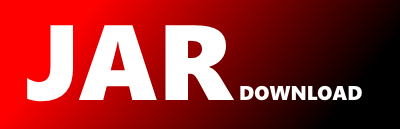
br.com.objectos.way.base.io.DataFileMeta Maven / Gradle / Ivy
/*
* Copyright 2013 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.base.io;
import java.io.File;
import java.io.IOException;
import java.nio.charset.Charset;
import java.util.List;
import br.com.objectos.core.io.Directory;
import org.joda.time.DateTime;
import com.google.common.base.Charsets;
import com.google.common.io.Files;
/**
* @author [email protected] (Marcio Endo)
*/
public class DataFileMeta {
final Charset charset = Charsets.UTF_8;
final Directory directory;
final String name;
final File file;
final DateTime lastModified;
DataFileMeta(Directory directory, String name) {
this.directory = directory;
this.name = name;
this.file = directory.fileAt(name);
long lastModified = file.lastModified();
this.lastModified = new DateTime(lastModified);
}
DataFileMeta(File file) {
this.directory = Directory.parentOf(file);
this.name = file.getName();
this.file = file;
long lastModified = file.lastModified();
this.lastModified = new DateTime(lastModified);
}
public Directory getDirectory() {
return directory;
}
public String getName() {
return name;
}
public String getPath() {
return file.getPath();
}
public DateTime getLastModified() {
return lastModified;
}
public byte[] toByteArray() throws IOException {
return Files.toByteArray(file);
}
@Override
public String toString() {
return file.toString();
}
public void copyFrom(File from) throws IOException {
Files.copy(from, file);
}
public List readLines() throws IOException {
return Files.readLines(file, charset);
}
public String readText() throws IOException {
return Files.toString(file, charset);
}
public String readTextOr(String or) {
try {
return Files.toString(file, charset);
} catch (IOException e) {
return or;
}
}
public String readTextOrEmpty() {
return readTextOr("");
}
public void write(String text) throws IOException {
Files.write(text, file, charset);
}
public void writeTo(File to) throws IOException {
Files.copy(file, to);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy