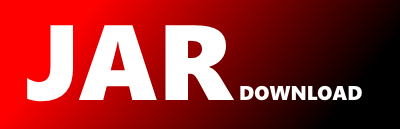
br.com.objectos.way.code.AnnotationValueInfo Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2014 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.code;
import br.com.objectos.way.core.auto.AutoFunctional;
import br.com.objectos.way.core.auto.AutoPojo;
import br.com.objectos.way.core.testing.Testable;
import com.google.common.base.Objects;
import com.google.common.base.Preconditions;
/**
* @author [email protected] (Marcio Endo)
*/
@AutoPojo
public abstract class AnnotationValueInfo implements Testable {
@AutoFunctional
abstract String name();
abstract AnnotationValueKind kind();
@AutoFunctional
abstract Object value();
AnnotationValueInfo() {
}
public static AnnotationValueInfoBuilder builder() {
return new AnnotationValueInfoBuilderPojo();
}
public > E getEnumValue(Class enumType) {
Preconditions.checkArgument(AnnotationValueKind.ENUM.equals(kind()));
EnumConstantInfo value = (EnumConstantInfo) value();
return value.getEnumValue(enumType);
}
public String getStringValue() {
Preconditions.checkArgument(AnnotationValueKind.STRING.equals(kind()));
return (String) value();
}
@Override
public String toString() {
return name() + "=" + value();
}
public boolean booleanValue() {
Preconditions.checkArgument(AnnotationValueKind.PRIMITIVE_BOOLEAN.equals(kind()));
return ((Boolean) value()).booleanValue();
}
public char charValue() {
Preconditions.checkArgument(AnnotationValueKind.PRIMITIVE_CHAR.equals(kind()));
return ((Character) value()).charValue();
}
public int intValue() {
Preconditions.checkArgument(AnnotationValueKind.PRIMITIVE_INT.equals(kind()));
return ((Integer) value()).intValue();
}
public SimpleTypeInfo simpleTypeInfoValue() {
Preconditions.checkArgument(AnnotationValueKind.TYPE.equals(kind()));
return (SimpleTypeInfo) value();
}
@Override
public final int hashCode() {
return Objects.hashCode(name(), kind(), value());
}
@Override
public final boolean equals(final Object obj) {
if (obj == this) {
return true;
}
if (obj instanceof AnnotationValueInfo) {
final AnnotationValueInfo that = (AnnotationValueInfo) obj;
return name().equals(that.name())
&& kind().equals(that.kind())
&& value().equals(that.value());
} else {
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy