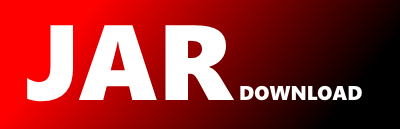
br.com.objectos.way.code.NameInfo Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2014 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.code;
import static com.google.common.collect.Lists.newArrayListWithCapacity;
import java.util.List;
import com.google.common.base.Joiner;
import com.google.common.base.Preconditions;
import com.google.common.base.Strings;
import com.google.common.collect.ImmutableList;
import com.squareup.javapoet.ClassName;
/**
* @author [email protected] (Marcio Endo)
*/
public class NameInfo {
private static final NameInfo EMPTY = new NameInfo(ImmutableList. of());
private final List nameList;
private NameInfo(List nameList) {
this.nameList = nameList;
}
public static NameInfo of() {
return EMPTY;
}
public static NameInfo of(String name) {
return Strings.isNullOrEmpty(name) ? EMPTY : new NameInfo(ImmutableList.of(name));
}
public static NameInfo nameOf(Iterable parts) {
List list = ImmutableList.copyOf(parts);
return new NameInfo(list);
}
public String getSimpleName() {
String res = "";
int size = nameList.size();
if (size > 0) {
res = nameList.get(size - 1);
}
return WayCode.stripGenerics(res);
}
@Override
public String toString() {
return Joiner.on('.').join(nameList);
}
public NameInfo prefix(String prefix) {
int size = nameList.size();
List copyList = newArrayListWithCapacity(size);
for (int i = 0; i < size; i++) {
String name = nameList.get(i);
if (i == size - 1) {
name = prefix + name;
}
copyList.add(name);
}
return new NameInfo(copyList);
}
public NameInfo suffix(String suffix) {
int size = nameList.size();
List copyList = newArrayListWithCapacity(size);
for (int i = 0; i < size; i++) {
String name = nameList.get(i);
if (i == size - 1) {
name = name + suffix;
}
copyList.add(name);
}
return new NameInfo(copyList);
}
@Override
public final int hashCode() {
return nameList.hashCode();
}
@Override
public final boolean equals(final Object obj) {
if (obj == this) {
return true;
}
if (obj instanceof NameInfo) {
final NameInfo that = (NameInfo) obj;
return nameList.equals(that.nameList);
} else {
return false;
}
}
boolean isQualifiedName() {
return nameList.size() > 1;
}
ClassName toClassName(PackageInfo packageInfo) {
int size = nameList.size();
Preconditions.checkArgument(size > 0);
String packageName = packageInfo.name();
String first = nameList.get(0);
String[] rest = nameList.subList(1, size).toArray(new String[] {});
return ClassName.get(packageName, first, rest);
}
NameInfo add(String name) {
List list = ImmutableList. builder()
.addAll(nameList)
.add(name)
.build();
return new NameInfo(list);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy