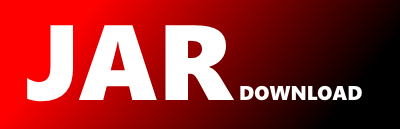
br.com.objectos.way.sql.ForeignKeyDefPojo Maven / Gradle / Ivy
/*
* Copyright 2015 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.sql;
import static com.google.common.collect.Lists.newArrayList;
import java.util.List;
import br.com.objectos.way.core.auto.AutoFunctional;
import br.com.objectos.way.core.util.WayIterables;
import br.com.objectos.way.sql.ForeignKeyDef.ForeignKeyDefOnDelete;
import br.com.objectos.way.sql.ForeignKeyDef.ForeignKeyDefOnUpdate;
import br.com.objectos.way.sql.ForeignKeyDef.ForeignKeyDefReferences;
import com.google.common.base.Optional;
/**
* @author [email protected] (Marcio Endo)
*/
abstract class ForeignKeyDefPojo
implements
ForeignKeyDef,
ForeignKeyDefOnDelete,
ForeignKeyDefOnUpdate,
ForeignKeyDefReferences {
private final TableDefPojo tableDef;
private final String name;
private final List foreignKeyPartDefList;
private ForeignKeyAction deleteAction;
private ForeignKeyAction updateAction;
public ForeignKeyDefPojo(TableDefPojo tableDef, String name) {
this.tableDef = tableDef;
this.name = name;
foreignKeyPartDefList = newArrayList();
}
public static ForeignKeyDefLevel1 level1(TableDefPojo tableDef, String name, ColumnRef columnRef) {
return new ForeignKeyDefLevel1(tableDef, name, columnRef);
}
@AutoFunctional
@Override
public ForeignKeyInfo toForeignKeyInfo() {
return ForeignKeyInfo.builder()
.name(Optional.fromNullable(name))
.foreignKeyPartList(foreignKeyPartList())
.deleteAction(Optional.fromNullable(deleteAction))
.updateAction(Optional.fromNullable(updateAction))
.build();
}
@Override
public TableInfo toTableInfo() {
return tableDef.toTableInfo();
}
@Override
public ConstraintDef constraint(String name) {
return tableDef.constraint(name);
}
@Override
public ForeignKeyDefOnDelete onDelete(ForeignKeyAction option) {
deleteAction = option;
return this;
}
@Override
public ForeignKeyDefOnUpdate onUpdate(ForeignKeyAction option) {
updateAction = option;
return this;
}
void addColumnRef(ColumnRef> columnRef) {
ForeignKeyPartDef partDef = new ForeignKeyPartDef(columnRef);
foreignKeyPartDefList.add(partDef);
}
void addReferencedColumnRef(int index, ColumnRef> columnRef) {
foreignKeyPartDefList.get(index).references(columnRef);
}
private List foreignKeyPartList() {
return WayIterables.from(foreignKeyPartDefList)
.transform(ForeignKeyPartDefToForeignKeyPart.get())
.toImmutableList();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy