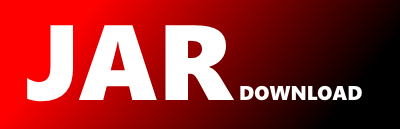
br.com.objectos.way.sql.ListSqlBuilderPojo Maven / Gradle / Ivy
package br.com.objectos.way.sql;
import com.google.common.base.Optional;
import java.util.List;
@javax.annotation.Generated("br.com.objectos.way.auto.pojo.AutoPojoProcessor")
final class ListSqlBuilderPojo
implements
ListSqlBuilder,
ListSqlBuilder.ListSqlBuilderSelectableList,
ListSqlBuilder.ListSqlBuilderTableReference,
ListSqlBuilder.ListSqlBuilderWhereCondition,
ListSqlBuilder.ListSqlBuilderGroupByInfo,
ListSqlBuilder.ListSqlBuilderHavingCondition,
ListSqlBuilder.ListSqlBuilderOrderByInfo {
private List selectableList;
private TableReference tableReference;
private Optional whereCondition;
private Optional groupByInfo;
private Optional havingCondition;
private Optional orderByInfo;
public ListSqlBuilderPojo() {
}
@Override
public ListSql build() {
return new ListSqlPojo(this);
}
@Override
public ListSqlBuilderSelectableList selectableList(List selectableList) {
if (selectableList == null) {
throw new NullPointerException();
}
this.selectableList = selectableList;
return this;
}
@Override
public ListSqlBuilderTableReference tableReference(TableReference tableReference) {
if (tableReference == null) {
throw new NullPointerException();
}
this.tableReference = tableReference;
return this;
}
@Override
public ListSqlBuilderWhereCondition whereCondition(Optional whereCondition) {
if (whereCondition == null) {
throw new NullPointerException();
}
this.whereCondition = whereCondition;
return this;
}
@Override
public ListSqlBuilderGroupByInfo groupByInfo(Optional groupByInfo) {
if (groupByInfo == null) {
throw new NullPointerException();
}
this.groupByInfo = groupByInfo;
return this;
}
@Override
public ListSqlBuilderHavingCondition havingCondition(Optional havingCondition) {
if (havingCondition == null) {
throw new NullPointerException();
}
this.havingCondition = havingCondition;
return this;
}
@Override
public ListSqlBuilderOrderByInfo orderByInfo(Optional orderByInfo) {
if (orderByInfo == null) {
throw new NullPointerException();
}
this.orderByInfo = orderByInfo;
return this;
}
List selectableList() {
return selectableList;
}
TableReference tableReference() {
return tableReference;
}
Optional whereCondition() {
return whereCondition;
}
Optional groupByInfo() {
return groupByInfo;
}
Optional havingCondition() {
return havingCondition;
}
Optional orderByInfo() {
return orderByInfo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy