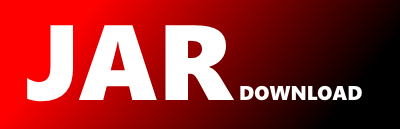
br.com.objectos.way.sql.SqlBuilderPojo Maven / Gradle / Ivy
/*
* Copyright 2014 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.sql;
import java.util.Iterator;
import com.google.common.base.Joiner;
import com.google.common.base.Preconditions;
/**
* @author [email protected] (Marcio Endo)
*/
class SqlBuilderPojo implements SqlBuilder {
private final StringBuilder builder = new StringBuilder();
private final Dialect dialect;
SqlBuilderPojo(Dialect dialect) {
this.dialect = dialect;
}
@Override
public String toString() {
return builder.toString();
}
@Override
public SqlBuilder append(char character) {
builder.append(character);
return this;
}
@Override
public SqlBuilder append(CanDecorateSqlBuilder element) {
Preconditions.checkNotNull(element);
return element.decorate(this);
}
@Override
public SqlBuilder append(String sql) {
Preconditions.checkNotNull(sql);
builder.append(sql);
return this;
}
public String build() {
return builder.toString();
}
@Override
public SqlBuilder endWhen() {
return this;
}
@Override
public SqlBuilder escape(String name) {
String escaped = dialect.escape(name);
return append(escaped);
}
@Override
public SqlBuilderJoiner on(char separator) {
return on(String.valueOf(separator));
}
@Override
public SqlBuilderJoiner on(String separator) {
Preconditions.checkNotNull(separator);
return new SqlBuilderJoinerPojo(separator);
}
@Override
public SqlBuilder qualifiedName(String first, String second) {
String qname = dialect.qualifiedName(first, second);
return append(qname);
}
@Override
public SqlBuilder space() {
return append(" ");
}
@Override
public SqlBuilderWhen when(boolean present) {
return present ? new SqlBuilderWhenPresent(this) : new SqlBuilderWhenAbsent(this);
}
private class SqlBuilderJoinerPojo implements SqlBuilderJoiner {
private final String separator;
public SqlBuilderJoinerPojo(String separator) {
this.separator = separator;
}
@Override
public SqlBuilder join(Iterable extends CanDecorateSqlBuilder> elements) {
SqlBuilder builder = SqlBuilderPojo.this;
Iterator extends CanDecorateSqlBuilder> iterator = elements.iterator();
if (iterator.hasNext()) {
CanDecorateSqlBuilder element = iterator.next();
element.decorate(builder);
while (iterator.hasNext()) {
element = iterator.next();
builder.append(separator);
element.decorate(builder);
}
}
return builder;
}
@Override
public SqlBuilder join(String[] strings) {
String sql = Joiner.on(separator).join(strings);
return append(sql);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy