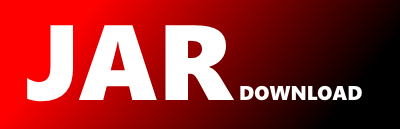
br.com.objectos.way.sql.WaySql Maven / Gradle / Ivy
/*
* Copyright 2014 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.sql;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.List;
import br.com.objectos.way.core.cache.TimeDuration;
import br.com.objectos.way.core.util.WayIterables;
import com.google.common.base.Function;
import com.google.common.base.Joiner;
import com.google.common.base.Optional;
import com.google.common.base.Predicate;
import com.google.common.base.Splitter;
import com.google.common.cache.Cache;
import com.google.common.cache.CacheBuilder;
/**
* @author [email protected] (Marcio Endo)
*/
public class WaySql {
private WaySql() {
}
public static Cache buildCache(
Optional expireAfterAccess,
Optional expireAfterWrite,
long maximumSize) {
CacheBuilder
© 2015 - 2025 Weber Informatics LLC | Privacy Policy