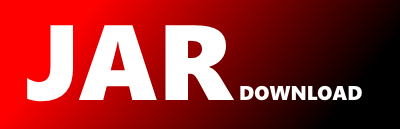
br.com.objectos.way.ui.Breadcrumb Maven / Gradle / Ivy
/*
* Copyright 2014 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.ui;
import static com.google.common.collect.Lists.newArrayList;
import java.util.List;
import org.codehaus.jackson.JsonGenerator;
import com.google.common.base.Function;
import com.google.common.base.Joiner;
import com.google.common.base.Predicates;
import com.google.common.collect.FluentIterable;
import com.google.common.collect.Lists;
import com.google.inject.Inject;
/**
* @author [email protected] (Marcio Endo)
*/
class Breadcrumb {
private final BaseUrl baseUrl;
private final List- items;
@Inject
public Breadcrumb(BaseUrl baseUrl) {
this(baseUrl, Lists.
- newArrayList());
}
private Breadcrumb(BaseUrl baseUrl, List
- items) {
this.baseUrl = baseUrl;
this.items = items;
}
public void add(String text, String link) {
items.add(new Item(text, link));
}
public void resume(Breadcrumb breadcrumb) {
items.addAll(breadcrumb.items);
}
List
get() {
return FluentIterable.from(items)
.transform(new ToCrumb())
.toList();
}
private class ToCrumb implements Function- {
private final List
links = newArrayList();
@Override
public Crumb apply(Item item) {
int index = links.size();
item.addTo(links);
return new Crumb(index, item.text, links);
}
}
private class Item {
final String text;
final String link;
public Item(String text, String link) {
this.text = text;
this.link = link;
}
public void addTo(List links) {
links.add(link);
}
}
private class Crumb implements IsJsonSerializable {
private final int index;
private final String text;
private final List links;
public Crumb(int index, String text, List links) {
this.index = index;
this.text = text;
this.links = FluentIterable.from(links)
.filter(Predicates.notNull())
.toList();
}
@Override
public void toJson(JsonGenerator jgen) {
WayUI.toJsonHelper(jgen)
.add("first", index == 0)
.add("text", text)
.add("url", getUrl())
.write();
}
private String getUrl() {
String url = Joiner.on("/").skipNulls().join(links);
return String.format("%s/%s", baseUrl, url);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy