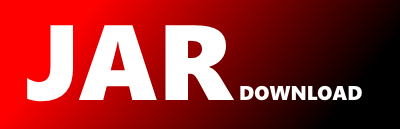
br.com.objectos.way.ui.PageRequestPojo Maven / Gradle / Ivy
/*
* Copyright 2014 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.ui;
import static com.google.common.collect.Sets.newHashSet;
import java.util.List;
import java.util.Set;
import javax.servlet.http.HttpServletRequest;
import com.google.common.base.Function;
import com.google.common.base.Optional;
import com.google.common.base.Predicate;
import com.google.common.base.Predicates;
import com.google.common.collect.FluentIterable;
import com.google.common.collect.ImmutableList;
import com.google.inject.Inject;
import com.google.inject.Provider;
import com.google.sitebricks.headless.Reply;
import com.google.sitebricks.headless.Request;
/**
* @author [email protected] (Marcio Endo)
*/
class PageRequestPojo implements PageRequest {
private final Method method;
private final Provider servletRequests;
private final Set methods;
private final Set conditions;
@Inject
public PageRequestPojo(Request request,
Provider servletRequests) {
this.method = Method.parse(request);
this.servletRequests = servletRequests;
this.methods = newHashSet();
this.conditions = newHashSet();
}
public PageRequestPojo(PageRequestBuilderPojo builder) {
method = builder.getMethod();
servletRequests = builder.getServletRequests();
methods = builder.getMethods();
conditions = builder.getConditions();
}
@SuppressWarnings("unchecked")
@Override
public T get(Class key) {
HttpServletRequest request = servletRequests.get();
return (T) request.getAttribute(key.getName());
}
@Override
public PageRequestPojo toPojo() {
return this;
}
PageRequest addAll(List requests) {
for (PageRequest request : requests) {
request.toPojo()
.addAllTo(this.conditions);
}
return this;
}
void addAllTo(Set thatConditions) {
thatConditions.addAll(this.conditions);
}
Optional> shouldUI() {
Optional condition = FluentIterable.from(conditions)
.filter(new PageConditionIsSatisfied())
.first();
List> optionals;
optionals = ImmutableList.of(condition);
Iterable conditions;
conditions = Optional.presentInstances(optionals);
return FluentIterable.from(conditions)
.filter(shouldCall())
.transform(ToReply.INSTANCE)
.filter(Predicates.notNull())
.first();
}
Predicate shouldCall() {
boolean result = methods.contains(method);
return result ?
Predicates. alwaysTrue() :
Predicates. alwaysFalse();
}
private class PageConditionIsSatisfied implements Predicate {
@Override
public boolean apply(PageCondition input) {
return input.isSatisfied(PageRequestPojo.this);
}
}
private enum ToReply implements Function> {
INSTANCE;
@Override
public Reply> apply(PageCondition input) {
return input.preReply();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy