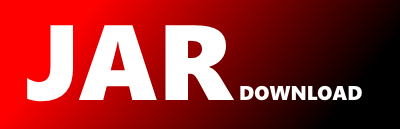
br.com.objectos.way.ui.ToJsonHelper Maven / Gradle / Ivy
/*
* Copyright 2014 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.ui;
import static com.google.common.collect.Lists.newArrayList;
import java.io.IOException;
import java.util.List;
import org.codehaus.jackson.JsonGenerationException;
import org.codehaus.jackson.JsonGenerator;
import com.google.common.base.Throwables;
/**
* @author [email protected] (Marcio Endo)
*/
public class ToJsonHelper {
private final JsonGenerator jgen;
private final List fields = newArrayList();
private JsonField current;
ToJsonHelper(JsonGenerator jgen) {
this.jgen = jgen;
}
public ToJsonHelper add(String fieldName, boolean value) {
this.current = new BooleanField(fieldName, value);
return addCurrentField();
}
public ToJsonHelper add(String fieldName, double value) {
this.current = new DoubleField(fieldName, value);
return addCurrentField();
}
public ToJsonHelper add(String fieldName, float value) {
this.current = new FloatField(fieldName, value);
return addCurrentField();
}
public ToJsonHelper add(String fieldName, int value) {
this.current = new IntegerField(fieldName, value);
return addCurrentField();
}
public ToJsonHelper add(String fieldName, long value) {
this.current = new LongField(fieldName, value);
return addCurrentField();
}
public ToJsonHelper add(String fieldName, Object value) {
this.current = new ObjectField(fieldName, value);
return addCurrentField();
}
public ToJsonHelper add(String fieldName, String value) {
this.current = new StringField(fieldName, value);
return addCurrentField();
}
public void write() {
try {
tryToWrite();
} catch (JsonGenerationException e) {
throw Throwables.propagate(e);
} catch (IOException e) {
throw Throwables.propagate(e);
}
}
private void tryToWrite() throws JsonGenerationException, IOException {
jgen.writeStartObject();
for (JsonField field : fields) {
field.write();
}
jgen.writeEndObject();
}
private ToJsonHelper addCurrentField() {
this.fields.add(current);
return this;
}
private class BooleanField extends JsonField {
private final boolean value;
public BooleanField(String fieldName, boolean value) {
super(fieldName);
this.value = value;
}
@Override
void write() throws JsonGenerationException, IOException {
jgen.writeBooleanField(fieldName, value);
}
}
private class DoubleField extends JsonField {
private final double value;
public DoubleField(String fieldName, double value) {
super(fieldName);
this.value = value;
}
@Override
void write() throws JsonGenerationException, IOException {
jgen.writeNumberField(fieldName, value);
}
}
private class FloatField extends JsonField {
private final float value;
public FloatField(String fieldName, float value) {
super(fieldName);
this.value = value;
}
@Override
void write() throws JsonGenerationException, IOException {
jgen.writeNumberField(fieldName, value);
}
}
private class IntegerField extends JsonField {
private final int value;
public IntegerField(String fieldName, int value) {
super(fieldName);
this.value = value;
}
@Override
void write() throws JsonGenerationException, IOException {
jgen.writeNumberField(fieldName, value);
}
}
private class LongField extends JsonField {
private final long value;
public LongField(String fieldName, long value) {
super(fieldName);
this.value = value;
}
@Override
void write() throws JsonGenerationException, IOException {
jgen.writeNumberField(fieldName, value);
}
}
private class ObjectField extends JsonField {
private final Object value;
public ObjectField(String fieldName, Object value) {
super(fieldName);
this.value = value;
}
@Override
void write() throws JsonGenerationException, IOException {
jgen.writeObjectField(fieldName, value);
}
}
private class StringField extends JsonField {
private final String value;
public StringField(String fieldName, String value) {
super(fieldName);
this.value = value;
}
@Override
void write() throws JsonGenerationException, IOException {
jgen.writeStringField(fieldName, value);
}
}
private abstract class JsonField {
final String fieldName;
public JsonField(String fieldName) {
this.fieldName = fieldName;
}
abstract void write() throws JsonGenerationException, IOException;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy