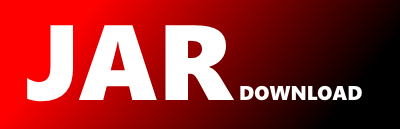
br.com.objectos.way.ui.UserInputConfigurePojo Maven / Gradle / Ivy
/*
* Copyright 2014 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.ui;
import static com.google.common.collect.Lists.newArrayList;
import java.util.List;
import br.com.objectos.way.ui.form.Errors;
import br.com.objectos.way.ui.form.FormResponse.Error;
import com.google.common.base.Optional;
import com.google.common.base.Preconditions;
/**
* @author [email protected] (Marcio Endo)
*/
class UserInputConfigurePojo
implements
UserInputConfigure,
UserInputConfigure.UserInputBuilderExecute {
private final List errors = newArrayList();
private final List formErrors = newArrayList();
private UserInputAction action;
private Context context;
private String redirectUrl;
@Override
public UserInputBuilderAddMessage addMessage(final String message) {
return new UserInputBuilderAddMessage() {
@Override
public UserInputConfigure toField(String fieldName) {
errors.add(Errors.toField(fieldName, message));
return UserInputConfigurePojo.this;
}
@Override
public UserInputConfigure toForm() {
formErrors.add(Errors.toForm(message));
return UserInputConfigurePojo.this;
}
};
}
@Override
public UserInputBuilderAddMessage addMessage(String template, Object... args) {
String message = String.format(template, args);
return addMessage(message);
}
@Override
public UserInputBuilderExecute execute(UserInputAction action) {
this.action = Preconditions.checkNotNull(action);
return this;
}
@Override
public UserInput reply() {
if (action != null) {
tryToExecute();
}
return UserInput.builder()
.context(Optional.fromNullable(context))
.errors(errors)
.formErrors(formErrors)
.redirectUrl(Optional.fromNullable(redirectUrl))
.build();
}
private void tryToExecute() {
if (errors.isEmpty() && formErrors.isEmpty()) {
try {
action.execute();
} catch (Throwable e) {
formErrors.add(Errors.toForm(e));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy