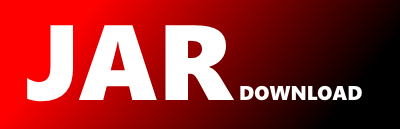
br.com.tecsinapse.dataio.txt.FileTxt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tecsinapse-data-io Show documentation
Show all versions of tecsinapse-data-io Show documentation
A simple way to work with CSV, XLSX? and TXT files
The newest version!
/*
* Tecsinapse Data Input and Output
*
* License: GNU Lesser General Public License (LGPL), version 3 or later
* See the LICENSE file in the root directory or .
*/
package br.com.tecsinapse.dataio.txt;
import java.util.ArrayList;
import java.util.List;
import br.com.tecsinapse.dataio.type.SeparatorType;
public class FileTxt {
private List> fields = new ArrayList<>();
private boolean endsWithSeparator = false;
public void addNewLine() {
fields.add(new ArrayList<>());
}
public void add(String content) {
add(FieldTxt.newBuilder()
.withContent(content)
.build());
}
public void addTabbed(String content) {
add(FieldTxt.newBuilder()
.withSeparator(SeparatorType.TAB)
.withContent(content)
.build());
}
public void addTabbedFixedSize(String content, int fixedSize) {
add(FieldTxt.newBuilder()
.withSeparator(SeparatorType.TAB)
.withContent(content)
.withFixedSize(fixedSize)
.build());
}
public void add(FieldTxt field) {
getLastLine().add(field);
}
public List getLastLine() {
return fields.get(getLastLineIndex());
}
public Integer getLastLineIndex() {
return fields.size() - 1;
}
public List> getFields() {
return fields;
}
public void setFields(List> fields) {
this.fields = fields;
}
public boolean isEndsWithSeparator() {
return endsWithSeparator;
}
public void setEndsWithSeparator(boolean endsWithSeparator) {
this.endsWithSeparator = endsWithSeparator;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy