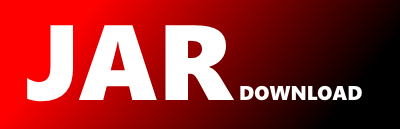
br.com.tecsinapse.dataio.util.CsvUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tecsinapse-data-io Show documentation
Show all versions of tecsinapse-data-io Show documentation
A simple way to work with CSV, XLSX? and TXT files
The newest version!
/*
* Tecsinapse Data Input and Output
*
* License: GNU Lesser General Public License (LGPL), version 3 or later
* See the LICENSE file in the root directory or .
*/
package br.com.tecsinapse.dataio.util;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import com.google.common.base.Strings;
import lombok.experimental.UtilityClass;
import br.com.tecsinapse.dataio.type.SeparatorType;
@UtilityClass
public class CsvUtil {
private final String DEFAULT_SEPARATOR_CHAR = SeparatorType.SEMICOLON.getSeparator();
private final String SEPARATOR = String.valueOf(DEFAULT_SEPARATOR_CHAR);
public static List processInputCSV(InputStream inputStream, Charset charset) throws IOException {
return processInputCSV(inputStream, true, charset);
}
public static List processCSV(InputStream inputStream, Charset charset) throws IOException {
return processInputCSV(inputStream, false, charset);
}
private static List processInputCSV(InputStream inputStream, boolean ignoreFirstLine, Charset charset) throws IOException {
List lines = new ArrayList<>();
try (BufferedReader br = new BufferedReader(new InputStreamReader(inputStream, charset))) {
String line;
if (ignoreFirstLine) {
line = br.readLine();
}
while ((line = br.readLine()) != null) {
if (!line.isEmpty() && line.split(SEPARATOR).length > 0) {
lines.add(line);
}
}
}
return lines;
}
public static String getColumnValue(String[] columns, int index) {
if (index >= columns.length || Strings.isNullOrEmpty(columns[index])) {
return null;
}
return columns[index].trim();
}
public static List simpleLine(Object[] fields) {
List list = new ArrayList<>();
if (fields != null && fields.length > 0) {
for (Object o : fields) {
if (o == null) {
list.add("");
} else {
list.add(o.toString());
}
}
}
return list;
}
public static File writeCsv(List> csv, String fileName, String chartsetName) throws IOException {
File file = new File(fileName + ".csv");
try (OutputStream o = new FileOutputStream(file)) {
write(csv, o, chartsetName);
}
return file;
}
public static void write(List> csv, OutputStream o, String chartsetName, char separator) throws IOException {
write(csv, o, chartsetName, String.valueOf(separator));
}
public static void write(List> csv, OutputStream o, String chartsetName, String separator) throws IOException {
try (OutputStreamWriter writer = new OutputStreamWriter(o, chartsetName)) {
for (List row : csv) {
StringBuilder line = new StringBuilder();
for (Iterator iter = row.iterator(); iter.hasNext(); ) {
final T next = iter.next();
String field = "";
if (next != null) {
field = String.valueOf(next).replace("\"", "\"\"");
if (field.indexOf(separator) > -1 || field.indexOf('"') > -1) {
field = '"' + field + '"';
}
}
line.append(field);
if (iter.hasNext()) {
line.append(separator);
}
}
line.append("\n");
writer.write(line.toString());
}
writer.flush();
}
}
public static void write(List> csv, OutputStream o, String chartsetName) throws IOException {
write(csv, o, chartsetName, DEFAULT_SEPARATOR_CHAR);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy