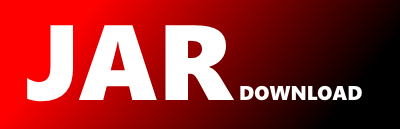
br.com.tecsinapse.dataio.util.FixedLengthFileUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tecsinapse-data-io Show documentation
Show all versions of tecsinapse-data-io Show documentation
A simple way to work with CSV, XLSX? and TXT files
The newest version!
/*
* Tecsinapse Data Input and Output
*
* License: GNU Lesser General Public License (LGPL), version 3 or later
* See the LICENSE file in the root directory or .
*/
package br.com.tecsinapse.dataio.util;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.List;
import lombok.experimental.UtilityClass;
@UtilityClass
public final class FixedLengthFileUtil {
public static List getLines(InputStream inputStream, boolean ignoreFirstLine, Charset charset) throws IOException {
return getLines(inputStream, ignoreFirstLine, 0, null, charset);
}
private static List getLines(InputStream inputStream, boolean ignoreFirstLine, int afterLine, String eofCharacter, Charset charset) throws IOException {
return getLines(inputStream, ignoreFirstLine, afterLine, 0, eofCharacter, charset);
}
public static List getLines(InputStream inputStream, boolean ignoreFirstLine, int afterLine, int ignoreLastLines, String eofCharacter, Charset charset) throws IOException {
List lines = new ArrayList<>();
try (BufferedReader br = new BufferedReader(new InputStreamReader(inputStream, charset))) {
String line;
afterLine += (ignoreFirstLine ? 1 : 0);
for (int i = 0; i < afterLine; i++) {
line = br.readLine();
}
line = br.readLine();
while (!isEof(line, eofCharacter)) {
if (!line.trim().isEmpty()) {
lines.add(line);
}
line = br.readLine();
}
}
if (ignoreLastLines > 0 && lines.size() >= ignoreLastLines) {
lines = lines.subList(0, lines.size() - ignoreLastLines);
}
return lines;
}
private static boolean isEof(String line, String eofCharacter) {
return line == null || (eofCharacter != null && line.contains(eofCharacter));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy