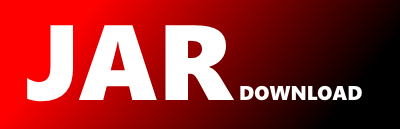
br.com.thiaguten.persistence.spi.provider.hibernate.HibernatePersistenceProvider Maven / Gradle / Ivy
/*
* #%L
* %%
* Copyright (C) 2015 - 2016 Thiago Gutenberg Carvalho da Costa.
* %%
* Redistribution and use in source and binary forms, with or without modification,
* are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* 3. Neither the name of the Thiago Gutenberg Carvalho da Costa. nor the names of its contributors
* may be used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
* IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT,
* INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
* BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF
* LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE
* OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED
* OF THE POSSIBILITY OF SUCH DAMAGE.
* #L%
*/
package br.com.thiaguten.persistence.spi.provider.hibernate;
import br.com.thiaguten.persistence.Persistable;
import org.hibernate.Criteria;
import org.hibernate.HibernateException;
import org.hibernate.Query;
import org.hibernate.Session;
import org.hibernate.criterion.Criterion;
import org.hibernate.criterion.Projections;
import org.hibernate.transform.ResultTransformer;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
/**
* Hibernate implementation of the PersistenceProvider.
*
* @author Thiago Gutenberg
*/
@SuppressWarnings("unchecked")
public abstract class HibernatePersistenceProvider implements HibernateCriteriaPersistenceProvider {
/**
* Get session
*
* @return session
*/
public abstract Session getSession();
/**
* Find an entity by its primary key.
*
* @param entityClazz the entity class
* @param pk the primary key
* @return the entity
*/
@Override
public , PK extends Serializable> T findById(Class entityClazz, PK pk) {
return (T) getSession().get(entityClazz, pk);
}
/**
* Load all entities.
*
* @param entityClazz the entity class
* @return the list of entities
*/
@Override
public > List findAll(Class entityClazz) {
return findByCriteria(entityClazz, null);
}
/**
* Load entities.
*
* @param entityClazz the entity class
* @param firstResult the value of first result
* @param maxResults the value of max result
* @return the list of entities
*/
@Override
public > List findAll(Class entityClazz, int firstResult, int maxResults) {
return findByCriteria(entityClazz, firstResult, maxResults, null);
}
/**
* Find by named query.
*
* @param entityClazz the entity class
* @param queryName the name of the query
* @param params the query parameters
* @return the list of entities
*/
@Override
public > List findByNamedQuery(Class entityClazz, String queryName, Object... params) {
Query hibernateQuery = getSession().getNamedQuery(queryName);
if (params != null) {
for (int i = 0; i < params.length; i++) {
hibernateQuery.setParameter(i + 1, params[i]);
}
}
return hibernateQuery.list();
}
/**
* Find by named query.
*
* @param entityClazz the entity class
* @param queryName the name of the query
* @param params the query parameters
* @return the list of entities
*/
@Override
public > List findByNamedQueryAndNamedParams(Class entityClazz, String queryName, Map params) {
Query hibernateQuery = getSession().getNamedQuery(queryName);
if (params != null && !params.isEmpty()) {
for (final Map.Entry param : params.entrySet()) {
hibernateQuery.setParameter(param.getKey(), param.getValue());
}
}
return hibernateQuery.list();
}
/**
* Find by query (JPQL/HQL, etc) and parameters.
*
* @param entityClazz the entity class
* @param query the typed query
* @param params the typed query parameters
* @return the list of entities
*/
@Override
public > List findByQueryAndNamedParams(Class entityClazz, String query, Map params) {
Query hibernateQuery = getSession().createQuery(query);
if (params != null && !params.isEmpty()) {
for (final Map.Entry param : params.entrySet()) {
hibernateQuery.setParameter(param.getKey(), param.getValue());
}
}
return hibernateQuery.list();
}
/**
* Count all entities.
*
* @param entityClazz the entity class
* @return the number of entities
*/
@Override
public > long countAll(Class entityClazz) {
return countByCriteria(entityClazz, Long.class, null);
}
/**
* Count by named query and parameters.
*
* @param resultClazz the number class
* @param queryName the named query
* @param params the named query parameters
* @return the count of entities
*/
@Override
public T countByNamedQueryAndNamedParams(Class resultClazz, String queryName, Map params) {
Query hibernateQuery = getSession().getNamedQuery(queryName);
if (params != null && !params.isEmpty()) {
for (final Map.Entry param : params.entrySet()) {
hibernateQuery.setParameter(param.getKey(), param.getValue());
}
}
return (T) hibernateQuery.uniqueResult();
}
/**
* Count by (JPQL/HQL, etc) and parameters.
*
* @param resultClazz the number class
* @param query the typed query
* @param params the typed query parameters
* @return the count of entities
*/
@Override
public T countByQueryAndNamedParams(Class resultClazz, String query, Map params) {
Query hibernateQuery = getSession().createQuery(query);
if (params != null && !params.isEmpty()) {
for (final Map.Entry param : params.entrySet()) {
hibernateQuery.setParameter(param.getKey(), param.getValue());
}
}
return (T) hibernateQuery.uniqueResult();
}
/**
* Save an entity.
*
* @param entity the entity to save
* @return the saved entity
*/
@Override
public > T save(T entity) {
Serializable id = entity.getId();
if (id != null) {
getSession().saveOrUpdate(entity);
} else {
id = getSession().save(entity);
}
entity = (T) findById(entity.getClass(), id);
return entity;
}
/**
* Update an entity.
*
* @param entity the entity to update
* @return the updated entity
*/
@Override
public > T update(T entity) {
return save(entity);
}
/**
* Delete an entity.
*
* @param entityClazz the entity class
* @param entity the entity to delete
*/
@Override
public > void delete(Class entityClazz, T entity) {
deleteByEntityOrId(entityClazz, entity, null);
}
/**
* Delete an entity.
*
* @param entityClazz the entity class
* @param pk primary key of the entity to delete
*/
@Override
public , PK extends Serializable> void deleteById(Class entityClazz, PK pk) {
deleteByEntityOrId(entityClazz, null, pk);
}
/**
* Find by criteria
*
* @param entityClazz the entity class
* @param criterions the criterions
* @param entity
* @return the list of entities
*/
@Override
public > List findByCriteria(Class entityClazz, List criterions) {
return findByCriteria(entityClazz, -1, -1, criterions);
}
/**
* Find by criteria
*
* @param entityClazz the entity class
* @param criterions the criterions
* @param firstResult first result
* @param maxResults max result
* @param entity
* @return the list of entities
*/
@Override
public > List findByCriteria(Class entityClazz, int firstResult, int maxResults, List criterions) {
return findByCriteria(entityClazz, false, firstResult, maxResults, criterions);
}
/**
* Find by criteria
*
* @param entityClazz the entity class
* @param criterions the criterions
* @param cacheable cacheable
* @param firstResult first result
* @param maxResults max result
* @param entity
* @return the list of entities
*/
@Override
public > List findByCriteria(Class entityClazz, boolean cacheable, int firstResult, int maxResults, List criterions) {
Criteria criteria = getSession().createCriteria(entityClazz);
if (criterions != null) {
for (Criterion c : criterions) {
criteria.add(c);
}
}
return criteriaRange(criteria, firstResult, maxResults).setCacheable(cacheable).list();
}
/**
* Find unique result by criteria
*
* @param entityClazz the entity class
* @param criterions the criterions
* @param entity
* @return the entity
*/
@Override
public > T findUniqueResultByCriteria(Class entityClazz, List criterions) {
return findUniqueResultByCriteria(entityClazz, false, criterions);
}
/**
* Find unique result by criteria
*
* @param entityClazz the entity class
* @param criterions the criterions
* @param cacheable cacheable
* @param entity
* @return the entity
*/
@Override
public > T findUniqueResultByCriteria(Class entityClazz, boolean cacheable, List criterions) {
Criteria criteria = getSession().createCriteria(entityClazz);
if (criterions != null) {
for (Criterion c : criterions) {
criteria.add(c);
}
}
return (T) criteria.setCacheable(cacheable).uniqueResult();
}
/**
* Count by criteria
*
* @param entityClazz the entity class
* @param resultClazz the result class
* @param criterions the criterions
* @param entity
* @param number pojo
* @return the count
*/
@Override
public , N extends Number> N countByCriteria(Class entityClazz, Class resultClazz, List criterions) {
return countByCriteria(entityClazz, resultClazz, Criteria.DISTINCT_ROOT_ENTITY, criterions);
}
/**
* Count by criteria
*
* @param entityClazz the entity class
* @param resultClazz the result class
* @param resultTransformer strategy for transforming query results
* @param criterions the criterions
* @param entity
* @param number pojo
* @return the count
*/
@Override
public , N extends Number> N countByCriteria(Class entityClazz, Class resultClazz, ResultTransformer resultTransformer, List criterions) {
Criteria criteria = getSession().createCriteria(entityClazz);
criteria.setProjection(Projections.rowCount());
if (criterions != null) {
for (Criterion c : criterions) {
criteria.add(c);
}
}
return (N) criteria.setResultTransformer(resultTransformer).uniqueResult();
}
private , PK extends Serializable> void deleteByEntityOrId(Class entityClazz, T entity, PK pk) {
if (pk == null && (entity == null || entity.getId() == null)) {
throw new HibernateException("Could not delete. ID is null.");
}
PK id = pk;
if (id == null) {
id = (PK) entity.getId();
}
T t = (T) getSession().load(entityClazz, id);
getSession().delete(t);
}
private Criteria criteriaRange(Criteria criteria, int firstResult, int maxResults) {
if (criteria != null) {
if (maxResults >= 0) {
criteria.setMaxResults(maxResults);
}
if (firstResult >= 0) {
criteria.setFirstResult(firstResult);
}
}
return criteria;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy