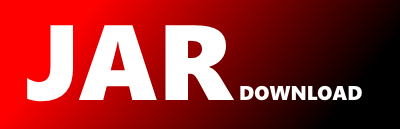
ca.bitcoco.jsk.redis.RedisCacheClient Maven / Gradle / Ivy
package ca.bitcoco.jsk.redis;
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
import redis.clients.jedis.JedisPoolConfig;
import java.time.Duration;
import java.util.Set;
public class RedisCacheClient {
final JedisPoolConfig poolConfig;
final JedisPool jedisPool;
public RedisCacheClient(String host) {
this.poolConfig = buildPoolConfig();
this.jedisPool = new JedisPool(this.poolConfig, host);
}
public boolean hasConnected() {
try (Jedis jedis = jedisPool.getResource()) {
return true;
} catch (Exception e) {
return false;
}
}
private JedisPoolConfig buildPoolConfig() {
final JedisPoolConfig poolConfig = new JedisPoolConfig();
poolConfig.setMaxTotal(128);
poolConfig.setMaxIdle(128);
poolConfig.setMinIdle(16);
poolConfig.setTestOnBorrow(true);
poolConfig.setTestOnReturn(true);
poolConfig.setTestWhileIdle(true);
poolConfig.setMinEvictableIdleTimeMillis(Duration.ofSeconds(60).toMillis());
poolConfig.setTimeBetweenEvictionRunsMillis(Duration.ofSeconds(30).toMillis());
poolConfig.setNumTestsPerEvictionRun(3);
poolConfig.setBlockWhenExhausted(true);
return poolConfig;
}
/**
* Set the string value as value of the key. The string can't be longer than 1073741824 bytes (1
* GB).
*
* @param key
* @param value
* @return Status code reply
*/
public String setString(String key, String value) {
try (Jedis jedis = jedisPool.getResource()) {
// NX|XX, NX -- Only set the key if it does not already exist. XX -- Only set the key if it already exist.
// EX|PX, expire time units: EX = seconds; PX = milliseconds
// expire time in the units of seconds, set default as 3600
return jedis.set(key, value, "NX", "EX", 3600);
}
}
/**
* Set the string value as value of the key. The string can't be longer than 1073741824 bytes (1
* GB).
*
* @param key
* @param value
* @param time expire time in the units of seconds
* @return Status code reply
*/
public String setString(String key, String value, final long time) {
try (Jedis jedis = jedisPool.getResource()) {
// NX|XX, NX -- Only set the key if it does not already exist. XX -- Only set the key if it already exist.
// EX|PX, expire time units: EX = seconds; PX = milliseconds
return jedis.set(key, value, "NX", "EX", time);
}
}
public String getString(String key) {
try (Jedis jedis = jedisPool.getResource()) {
return jedis.get(key);
}
}
public String getString(String key, String defaultString) {
try (Jedis jedis = jedisPool.getResource()) {
if (jedis.get(key) != null) {
return jedis.get(key);
} else {
return defaultString;
}
}
}
public Long deleteCache(String key) {
try (Jedis jedis = jedisPool.getResource()) {
return jedis.del(key);
}
}
public Long deleteCache(String... keys) {
try (Jedis jedis = jedisPool.getResource()) {
return jedis.del(keys);
}
}
/**
* Set the string value as value of the key. The string can't be longer than 1073741824 bytes (1
* GB).
*
* @param prefix could be * for all
* @return set of keys
*/
public Set getKeys(String prefix) {
try (Jedis jedis = jedisPool.getResource()) {
return jedis.keys(prefix + "*");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy