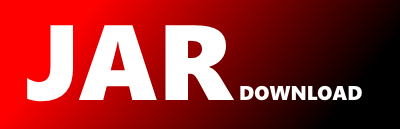
ca.bitcoco.jsk.wechat.WeChatClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsk-starter Show documentation
Show all versions of jsk-starter Show documentation
Common service for bitcoco usage
package ca.bitcoco.jsk.wechat;
import ca.bitcoco.jsk.http.HttpClient;
import ca.bitcoco.jsk.http.HttpClientResponse;
import ca.bitcoco.jsk.http.HttpMethod;
import org.json.JSONObject;
import java.util.HashMap;
import java.util.Map;
public class WeChatClient {
//state 1(open):微信开放平台() 2(public): 微信公众平台
//WX_PUBLIC_APP_ID / WX_PUBLIC_APP_SECRET 微信公众平台(用于微信内API,微信APP内公众号服务,微信分享以及微信Browser内授权登录第三方)
//WX_OPEN_APP_ID / WX_OPEN_APP_SECRET 微信开放平台(用于微信外API,如第三方扫码登录)@Getter
private String WX_OPEN_APP_ID;
private String WX_OPEN_APP_SECRET;
private String WX_PUBLIC_APP_ID;
private String WX_PUBLIC_APP_SECRET;
public WeChatClient(String WX_OPEN_APP_ID, String WX_OPEN_APP_SECRET, String WX_PUBLIC_APP_ID, String WX_PUBLIC_APP_SECRET) {
this.WX_OPEN_APP_ID = WX_OPEN_APP_ID;
this.WX_OPEN_APP_SECRET = WX_OPEN_APP_SECRET;
this.WX_PUBLIC_APP_ID = WX_PUBLIC_APP_ID;
this.WX_PUBLIC_APP_SECRET = WX_PUBLIC_APP_SECRET;
}
public String getWX_OPEN_APP_ID() {
return WX_OPEN_APP_ID;
}
public String getWX_PUBLIC_APP_ID() {
return WX_PUBLIC_APP_ID;
}
public Map authCode(String code, String state) throws Exception {
JSONObject accessTokenResult = this.fetchWXLoginResponse(code, state);
String accessToken = accessTokenResult.get("access_token") != null ? (String) accessTokenResult.get("access_token") : "";
String userOpenId = accessTokenResult.get("openid") != null ? (String) accessTokenResult.get("openid") : "";
String refreshToken = accessTokenResult.get("refresh_token") != null ? (String) accessTokenResult.get("refresh_token") : "";
if (accessToken.isEmpty() || userOpenId.isEmpty()) {
throw new Exception("wechat auth failed, can not get access_token");
}
JSONObject userDataResponse = this.fetchWXLoginUserResponse(accessToken, userOpenId);
if (userDataResponse != null) {
Map response = new HashMap<>();
String openId = userDataResponse.get("unionid") != null ? userDataResponse.get("unionid").toString() : null;
String name = userDataResponse.get("nickname") != null ? userDataResponse.get("nickname").toString() : "";
String pictureUrl = userDataResponse.get("headimgurl") != null ? userDataResponse.get("headimgurl").toString() : "";
String locale = userDataResponse.get("country") != null ? userDataResponse.get("country").toString() : "";
response.put("openId", openId);
response.put("email", "");
response.put("name", name);
response.put("pictureUrl", pictureUrl);
response.put("locale", locale);
response.put("lastName", "");
response.put("firstName", name);
return response;
} else {
return null;
}
}
//based on state, get access_token from different places
//state 1(open):微信开放平台() 2(public): 微信公众平台
//WX_PUBLIC_APP_ID / WX_PUBLIC_APP_SECRET 微信公众平台(用于微信内API,微信APP内公众号服务,微信分享以及微信Browser内授权登录第三方)
//WX_OPEN_APP_ID / WX_OPEN_APP_SECRET 微信开放平台(用于微信外API,如第三方扫码登录)
private JSONObject fetchWXLoginResponse(String code, String state) throws Exception {
try {
String appId = state.equalsIgnoreCase("open") || state.equalsIgnoreCase("1") ? WX_OPEN_APP_ID : WX_PUBLIC_APP_ID;
String secret = state.equalsIgnoreCase("open") || state.equalsIgnoreCase("1") ? WX_OPEN_APP_SECRET : WX_PUBLIC_APP_SECRET;
String url = "https://api.weixin.qq.com/sns/oauth2/access_token?appid=" + appId + "&secret=" + secret + "&code=" + code + "&grant_type=authorization_code";
HttpClientResponse response = new HttpClient()
.URL(url)
.method(HttpMethod.GET)
.send();
JSONObject result = new JSONObject(response.getBody());
if (result.get("errcode") != null) {
long errcode = Long.parseLong(result.get("errcode").toString());
String errmsg = result.get("errmsg") != null ? (String) result.get("errmsg") : "";
throw new Exception(errcode + " " + errmsg);
}
return result;
} catch (Exception e) {
throw new Exception("wechat fetch public access token failed, " + e.getLocalizedMessage());
}
}
private JSONObject fetchWXLoginUserResponse(String accessToken, String openId) throws Exception {
String url = "https://api.weixin.qq.com/sns/userinfo?access_token=" + accessToken + "&openid=" + openId;
HttpClientResponse response = new HttpClient()
.URL(url)
.method(HttpMethod.GET)
.send();
JSONObject result = new JSONObject(response.getBody());
if (result.get("errcode") != null) {
String errcode = (String) result.get("errcode");
String errmsg = (String) result.get("errmsg");
throw new Exception("wechat auth get user info failed, " + errcode + " " + errmsg);
} else {
return result;
}
}
/**
* 从服务端获取wx public token 并保存在redis
*/
public String fetchWXPublicAccessToken() throws Exception {
try {
String grantType = "client_credential";
String appId = WX_PUBLIC_APP_ID;
String secret = WX_PUBLIC_APP_SECRET;
String url = "https://api.weixin.qq.com/cgi-bin/token?grant_type=" + grantType + "&appid=" + appId + "&secret=" + secret;
HttpClientResponse response = new HttpClient()
.URL(url)
.method(HttpMethod.GET)
.send();
JSONObject result = new JSONObject(response.getBody());
if (result.get("errcode") != null) {
long errcode = Long.parseLong(result.get("errcode").toString());
String errmsg = result.get("errmsg") != null ? (String) result.get("errmsg") : "";
throw new Exception( errcode + " " + errmsg);
} else {
JSONObject data = new JSONObject((String) result.get("data"));
return (String) data.get("access_token");
}
} catch (Exception e) {
throw new Exception("wechat fetch public access token failed, " + e.getLocalizedMessage());
}
}
/**
* 从服务端获取ticket
*/
public String fetchWXPublicTicket(String token) throws Exception {
try {
String requestType = "jsapi";
String url = "https://api.weixin.qq.com/cgi-bin/ticket/getticket?access_token=" + token + "&type=" + requestType;
HttpClientResponse response = new HttpClient()
.URL(url)
.method(HttpMethod.GET)
.send();
JSONObject result = new JSONObject(response.getBody());
if (result.get("errcode") != null) {
long errcode = Long.parseLong(result.get("errcode").toString());
String errmsg = result.get("errmsg") != null ? (String) result.get("errmsg") : "";
throw new Exception(errcode + " " + errmsg);
} else {
JSONObject data = new JSONObject((String) result.get("data"));
return (String) data.get("ticket");
}
} catch (Exception e) {
throw new Exception("wechat fetch ticket failed, " + e.getLocalizedMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy