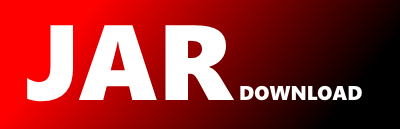
ca.bitcoco.jsk.http.HttpClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsk-starter Show documentation
Show all versions of jsk-starter Show documentation
Common service for bitcoco usage
The newest version!
package ca.bitcoco.jsk.http;
import org.apache.http.HttpEntity;
import org.apache.http.NameValuePair;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.*;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
public class HttpClient {
private RequestConfig requestConfig;
private HttpMethod method;
private HashMap headers;
private String url;
private String JSONBody;
private String name;
private HashMap postParams;
private int retry;
private final int defaultCancelTimeout = 3000; // 3s
private boolean recordMetric = false;
private String metricPath;
// default cancel timeout as 3 seconds
public HttpClient(String name) {
this.name = name;
this.method = HttpMethod.GET;
this.headers = new HashMap<>();
this.retry = 0;
this.url = "";
this.recordMetric = false;
this.metricPath = "";
this.requestConfig = RequestConfig.custom()
.setConnectionRequestTimeout(defaultCancelTimeout)
.setConnectTimeout(defaultCancelTimeout)
.setSocketTimeout(defaultCancelTimeout)
.build();
}
public HttpClient cancelTimeout(int milliseconds){
this.requestConfig = RequestConfig.custom()
.setConnectionRequestTimeout(milliseconds)
.setConnectTimeout(milliseconds)
.setSocketTimeout(milliseconds)
.build();
return this;
}
public HttpClient recordMetric(String metricPath) {
this.recordMetric = true;
this.metricPath = metricPath;
return this;
}
public HttpClient URL(String url) {
this.url = url;
return this;
}
public HttpClient addHeader(HttpHeader name, String value) {
this.headers.put(name.key(), value);
return this;
}
public HttpClient method(HttpMethod method) {
this.method = method;
return this;
}
public HttpClient JSONBody(String body) {
this.JSONBody = body;
return this;
}
public HttpClient retry(int count) {
if (count > 0) {
this.retry = count;
}
return this;
}
public HttpClient postParams(HashMap postParams) {
this.postParams = postParams;
return this;
}
public HttpClient requestConfig(RequestConfig requestConfig) {
this.requestConfig = requestConfig;
return this;
}
private HttpRequestBase getRequest() throws UnsupportedEncodingException {
HttpRequestBase request;
switch (method) {
case GET:
request = new HttpGet(this.url);
break;
case POST:
HttpPost post = new HttpPost(this.url);
if (this.JSONBody != null) {
post.setEntity(new StringEntity(this.JSONBody));
headers.put("Content-Type", "application/json");
} else if (this.postParams != null) {
List urlParameters = new ArrayList<>();
this.postParams.forEach((k, v) -> {
urlParameters.add(new BasicNameValuePair(k, v));
});
post.setEntity(new UrlEncodedFormEntity(urlParameters));
}
request = post;
break;
case PUT:
HttpPut put = new HttpPut(this.url);
if (this.JSONBody != null) {
put.setEntity(new StringEntity(this.JSONBody));
headers.put("Content-Type", "application/json");
} else if (this.postParams != null) {
List urlParameters = new ArrayList<>();
this.postParams.forEach((k, v) -> {
urlParameters.add(new BasicNameValuePair(k, v));
});
put.setEntity(new UrlEncodedFormEntity(urlParameters));
}
request = put;
break;
case PATCH:
HttpPatch patch = new HttpPatch(this.url);
if (this.JSONBody != null) {
patch.setEntity(new StringEntity(this.JSONBody));
headers.put("Content-Type", "application/json");
} else if (this.postParams != null) {
List urlParameters = new ArrayList<>();
this.postParams.forEach((k, v) -> {
urlParameters.add(new BasicNameValuePair(k, v));
});
patch.setEntity(new UrlEncodedFormEntity(urlParameters));
}
request = patch;
break;
case DELETE:
request = new HttpDelete(this.url);
break;
default:
request = new HttpGet(this.url);
}
headers.put("Accept", "*/*");
headers.put("Accept-Encoding", "gzip, deflate, br");
headers.put("Connection", "keep-alive");
headers.forEach(request::addHeader);
request.setConfig(this.requestConfig);
return request;
}
public HttpClientResponse send() throws Exception {
if (this.url == null) {
throw new Exception("HttpClient: Missing URL");
}
long startTime = System.currentTimeMillis();
try (CloseableHttpClient httpClient = HttpClients.createDefault()) {
int count = 0;
do {
try (CloseableHttpResponse response = httpClient.execute(this.getRequest())) {
long endTime = System.currentTimeMillis();
HttpClientResponse customResponse = new HttpClientResponse();
// Get HttpResponse Status
customResponse.setDuration(endTime - startTime);
customResponse.setStatusCode(response.getStatusLine().getStatusCode()); // 200
customResponse.setProtocolVersion(response.getProtocolVersion().toString()); // HTTP/1.1
customResponse.setReasonPhrase(response.getStatusLine().getReasonPhrase()); // OK
HttpEntity entity = response.getEntity();
if (this.recordMetric) {
HttpUtils.RecordHttpClientRequestDurationMetric(this.name,
this.method.key(),
this.metricPath,
customResponse.getStatusCode(),
customResponse.getDuration());
}
if (entity != null) {
// return it as a String
customResponse.setBody(EntityUtils.toString(entity));
return customResponse;
} else {
count++;
}
} catch (Exception ex) {
count++;
if (count > this.retry) {
throw ex;
}
}
} while (count <= this.retry);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy