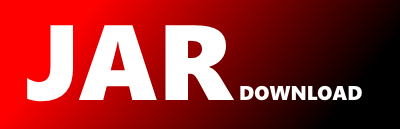
ca.bitcoco.jsk.operation.Operation Maven / Gradle / Ivy
package ca.bitcoco.jsk.operation;
import ca.bitcoco.jsk.globalConfig.Versioning;
import ca.bitcoco.jsk.operation.log.Logger;
import io.opentelemetry.api.GlobalOpenTelemetry;
import io.opentelemetry.api.OpenTelemetry;
import io.opentelemetry.api.common.Attributes;
import io.opentelemetry.api.trace.Span;
import io.opentelemetry.api.trace.Tracer;
public class Operation {
Logger logger;
Tracer tracer;
String operationName;
Span span;
String traceId;
Long startTime;
boolean recordLatency;
public Operation(String operationName) {
this.operationName = operationName;
OpenTelemetry openTelemetry = GlobalOpenTelemetry.get();
this.tracer = openTelemetry.getTracer("", "1.0.0");
}
public Operation(String operationName, boolean recordLatency) {
this.operationName = operationName;
this.tracer = GlobalOpenTelemetry.getTracerProvider().get("");
this.recordLatency = recordLatency;
}
public Span start() {
span = tracer.spanBuilder(this.operationName).startSpan();
setDefaultLabel(span);
traceId = span.getSpanContext().getTraceId();
startTime = System.currentTimeMillis();
return span;
}
private void setDefaultLabel(Span span) {
if (span != null) {
span.setAttribute("env", Versioning.env);
span.setAttribute("region", Versioning.region);
span.setAttribute("version", Versioning.version);
}
}
public void setLabel(String key, String value) {
if (span != null) {
span.setAttribute(key, value);
}
}
public void setLabel(String key, double value) {
if (span != null) {
span.setAttribute(key, value);
}
}
public void setLabel(String key, long value) {
if (span != null) {
span.setAttribute(key, value);
}
}
public void setLabel(String key, boolean value) {
if (span != null) {
span.setAttribute(key, value);
}
}
public void finish() {
if (span != null) {
span.setAttribute("success", true);
span.end();
}
if (recordLatency) {
long finish = System.currentTimeMillis();
long timeElapsed = finish - this.startTime;
GlobalMetric.RecordOperationLatency(this.operationName, timeElapsed, true);
}
}
public void finish(boolean success) {
if (span != null) {
span.setAttribute("success", success);
span.end();
}
if (recordLatency) {
long finish = System.currentTimeMillis();
long timeElapsed = finish - this.startTime;
GlobalMetric.RecordOperationLatency(this.operationName, timeElapsed, success);
}
}
public void finish(Exception ex) {
if (span != null) {
span.setAttribute("success", false);
span.addEvent("error", Attributes.builder().put("message", ex.getMessage()).build());
span.end();
}
if (recordLatency) {
long finish = System.currentTimeMillis();
long timeElapsed = finish - this.startTime;
GlobalMetric.RecordOperationLatency(this.operationName, timeElapsed, false);
}
}
public Logger logger(Class thisClass) {
if (this.logger == null) {
this.logger = new Logger(thisClass.getName());
}
if (this.span != null) {
this.logger.setSpan(this.span);
}
if (this.traceId != null) {
this.logger.setTraceId(this.traceId);
}
this.logger.setOperationName(operationName);
return this.logger;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy