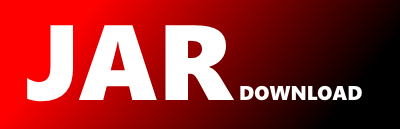
ca.carleton.gcrc.couch.command.Main Maven / Gradle / Ivy
package ca.carleton.gcrc.couch.command;
import java.io.File;
import java.io.PrintStream;
import java.util.ArrayList;
import java.util.List;
import java.util.Vector;
import org.apache.log4j.Level;
import org.apache.log4j.Logger;
import org.apache.log4j.PatternLayout;
import org.apache.log4j.PropertyConfigurator;
import org.apache.log4j.WriterAppender;
import ca.carleton.gcrc.couch.command.impl.PathComputer;
public class Main {
//final static private org.slf4j.Logger logger = LoggerFactory.getLogger(Main.class.getClass());
static private List allCommands = null;
synchronized static public List getCommands(){
if( null == allCommands ) {
allCommands = new Vector();
allCommands.add( new CommandHelp() );
allCommands.add( new CommandCreate() );
allCommands.add( new CommandConfig() );
allCommands.add( new CommandUpdate() );
allCommands.add( new CommandUpdateUser() );
allCommands.add( new CommandRun() );
allCommands.add( new CommandDump() );
allCommands.add( new CommandRestore() );
allCommands.add( new CommandUpgrade() );
allCommands.add( new CommandVersion() );
allCommands.add( new CommandAddSchema() );
allCommands.add( new CommandUpdateSchema() );
}
return allCommands;
}
static public void main(String[] args) {
GlobalSettings globalSettings = null;
try {
List arguments = new ArrayList(args.length);
for(String arg : args){
arguments.add(arg);
}
globalSettings = new GlobalSettings();
Main app = new Main();
app.execute(globalSettings, arguments);
System.exit(0);
} catch(Exception e) {
//logger.error("Error: "+e.getMessage(),e);
PrintStream err = System.err;
if( null != globalSettings ) {
err = globalSettings.getErrStream();
}
if( null != globalSettings
&& globalSettings.isDebug() ){
e.printStackTrace(err);
} else {
err.print("Error: "+e.getMessage());
err.println();
int limit = 10;
Throwable cause = e.getCause();
while(null != cause && limit > 0) {
err.print("Caused by: "+cause.getMessage());
err.println();
cause = cause.getCause();
--limit;
}
}
// Error
System.exit(1);
}
}
public void execute(GlobalSettings globalSettings, List args) throws Exception {
Options options = new Options();
options.parseOptions(args);
List arguments = options.getArguments();
// Process global options
processGlobalOptions(globalSettings, options);
// Default log4j configuration
{
Logger rootLogger = Logger.getRootLogger();
rootLogger.setLevel(Level.ERROR);
rootLogger.addAppender(new WriterAppender(new PatternLayout("%d{ISO8601}[%-5p]: %m%n"),globalSettings.getErrStream()));
}
// Compute needed file paths
{
File installDir = PathComputer.computeInstallDir();
globalSettings.setInstallDir( installDir );
}
// Find out command
if( arguments.size() < 1 ){
throw new Exception("No command provided. Try 'help'.");
}
String commandKeyword = arguments.get(0);
for(Command command : getCommands()){
if( command.matchesKeyword(commandKeyword) ) {
// Found the command in question
// Check options for this command
String[] expectedOptions = command.getExpectedOptions();
options.validateExpectedOptions(expectedOptions);
// Execute
performCommand(
command
,globalSettings
,options
);
return;
}
}
// At this point the command was not found
throw new Exception("Can not understand command: "+commandKeyword);
}
private void processGlobalOptions(
GlobalSettings globalSettings
,Options options
) throws Exception {
// Atlas directory
String atlasDirStr = options.getAtlasDir();
if( null != atlasDirStr ){
File atlasDir = PathComputer.computeAtlasDir(atlasDirStr);
globalSettings.setAtlasDir(atlasDir);
}
// Debug
Boolean debug = options.getDebug();
if( null != debug ){
if( debug.booleanValue() ){
globalSettings.setDebug(true);
}
}
}
private void performCommand(Command command, GlobalSettings gs, Options options) throws Exception {
if( command.requiresAtlasDir() ){
if( null == gs.getAtlasDir() ) {
// Use current directory
gs.setAtlasDir( PathComputer.computeAtlasDir(null) );
}
// Verify that this is valid a nunaliit directory
// Check that atlas directory exists
File atlasDir = gs.getAtlasDir();
if( false == atlasDir.exists() ){
throw new Exception("Atlas directory not found: "+atlasDir.getAbsolutePath());
}
if( false == atlasDir.isDirectory() ){
throw new Exception("Atlas is not a directory: "+atlasDir.getAbsolutePath());
}
// Check nunaliit.properties file
{
File propFile = new File(atlasDir,"config/nunaliit.properties");
if( false == propFile.exists()
|| false == propFile.isFile() ) {
throw new Exception("Directory does not appear to contain an atlas: "+atlasDir.getAbsolutePath());
}
}
}
// Configure log4j
{
// Try to load config/log4j.properties
File atlasDir = gs.getAtlasDir();
File log4jConfFile = new File(atlasDir, "config/log4j.properties");
if( log4jConfFile.exists()
&& log4jConfFile.isFile() ) {
PropertyConfigurator.configure(log4jConfFile.getAbsolutePath());
}
}
command.runCommand(gs ,options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy