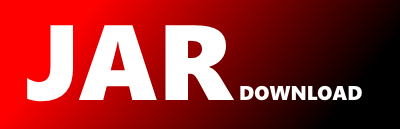
ca.carleton.gcrc.couch.command.dump.RestoreMain Maven / Gradle / Ivy
package ca.carleton.gcrc.couch.command.dump;
import java.io.File;
import java.io.PrintStream;
import java.util.ArrayList;
import java.util.List;
import java.util.Stack;
import ca.carleton.gcrc.couch.app.DbRestoreListener;
import ca.carleton.gcrc.couch.app.DbRestoreProcess;
import ca.carleton.gcrc.couch.client.CouchDb;
import ca.carleton.gcrc.couch.command.impl.RestoreListener;
public class RestoreMain {
static public void main(String[] args) {
DumpSettings dumpSettings = null;
try {
List arguments = new ArrayList(args.length);
for(String arg : args){
arguments.add(arg);
}
dumpSettings = new DumpSettings(DumpSettings.Type.RESTORE);
RestoreMain app = new RestoreMain();
app.execute(dumpSettings, arguments);
System.exit(0);
} catch(Exception e) {
PrintStream err = System.err;
if( null != dumpSettings ) {
err = dumpSettings.getErrStream();
}
if( null != dumpSettings
&& dumpSettings.isDebug() ){
e.printStackTrace(err);
} else {
err.print("Error: "+e.getMessage());
err.println();
int limit = 10;
Throwable cause = e.getCause();
while(null != cause && limit > 0) {
err.print("Caused by: "+cause.getMessage());
err.println();
cause = cause.getCause();
--limit;
}
}
// Error
System.exit(1);
}
}
public void execute(DumpSettings dumpSettings, List args) throws Exception {
// Turn arguments into a stack
Stack argumentStack = new Stack();
for(int i=args.size()-1; i>=0; --i){
argumentStack.push( args.get(i) );
}
// Process global options
dumpSettings.parseCommandLineArguments(argumentStack);
// Check for help
if( dumpSettings.isHelpRequested() ) {
help( dumpSettings.getOutStream() );
return;
}
// Ask the user about the missing options
dumpSettings.acceptUserOptions();
// Check that dump dir exists
File dumpDir = dumpSettings.getDumpDir();
if( null == dumpDir ) {
throw new Exception("--dump-dir must be specified");
}
// Get CouchDb client
CouchDb couchDb = dumpSettings.createCouchDb();
// Create restore process
DbRestoreListener listener = new RestoreListener(dumpSettings.getOutStream());
DbRestoreProcess restoreProcess = new DbRestoreProcess(couchDb, dumpDir);
restoreProcess.setListener(listener);
List docIds = dumpSettings.getDocIds();
if( docIds.size() < 1 ) {
restoreProcess.setAllDocs(true);
} else {
for(String docId : docIds) {
restoreProcess.addDocId(docId);
}
}
restoreProcess.restore();
}
private void help(PrintStream ps) {
ps.println("couch-restore - Help");
ps.println();
ps.println("Command Syntax:");
ps.println(" couch-restore [
© 2015 - 2025 Weber Informatics LLC | Privacy Policy