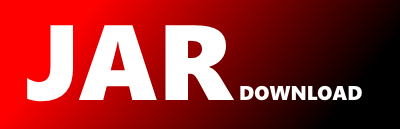
ca.carleton.gcrc.couch.command.impl.SkeletonDocumentsDetector Maven / Gradle / Ivy
package ca.carleton.gcrc.couch.command.impl;
import java.io.File;
import java.util.Collection;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.Vector;
import org.json.JSONObject;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import ca.carleton.gcrc.couch.client.CouchDb;
import ca.carleton.gcrc.couch.client.CouchDesignDocument;
import ca.carleton.gcrc.couch.client.CouchQuery;
import ca.carleton.gcrc.couch.client.CouchQueryResults;
import ca.carleton.gcrc.couch.command.GlobalSettings;
public class SkeletonDocumentsDetector {
final protected Logger logger = LoggerFactory.getLogger(this.getClass());
private GlobalSettings gs;
private CouchDb couchDb;
public SkeletonDocumentsDetector(
CouchDb couchDb
,GlobalSettings gs
){
this.couchDb = couchDb;
this.gs = gs;
}
public List getSkeletonDocIds() throws Exception {
Set docIds = new HashSet();
// Add all documents in "docs" directory
{
logger.debug("Obtain list of documents in 'docs'");
File atlasDir = gs.getAtlasDir();
File docsDir = new File(atlasDir, "docs");
Collection skeletonDocIds = listDocumentsFromDir(gs,docsDir);
docIds.addAll(skeletonDocIds);
}
// Add documents marked as skeleton
{
logger.debug("Obtain list of documents from skeleton-docs view");
CouchDesignDocument designDoc = couchDb.getDesignDocument("atlas");
CouchQuery query = new CouchQuery();
query.setViewName("skeleton-docs");
CouchQueryResults results = designDoc.performQuery(query);
List rows = results.getRows();
for(JSONObject row : rows){
String docId = row.getString("id");
docIds.add(docId);
}
}
// Remove documents provided by nunaliit
logger.debug("Remove list of documents provided by Nunaliit");
{
File docsDir = PathComputer.computeInitializeDocsDir(gs.getInstallDir());
Collection nunaliitDocIds = listDocumentsFromDir(gs,docsDir);
docIds.removeAll(nunaliitDocIds);
}
{
File docsDir = PathComputer.computeUpdateDocsDir(gs.getInstallDir());
Collection nunaliitDocIds = listDocumentsFromDir(gs,docsDir);
docIds.removeAll(nunaliitDocIds);
}
return new Vector(docIds);
}
private Collection listDocumentsFromDir(
GlobalSettings gs
,File docsDir
) throws Exception {
Map docIdsToFiles = FileUtils.listDocumentsFromDir(gs, docsDir);
return docIdsToFiles.keySet();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy