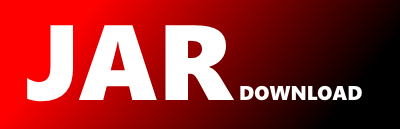
jas.ClassEnv Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jasmin Show documentation
Show all versions of jasmin Show documentation
Java Assembler Interface for the Soot framework
package jas;
import java.io.*;
import java.util.Hashtable;
import java.util.Enumeration;
import java.util.Vector;
import java.util.Iterator;
/**
* This is the place where all information about the class to
* be created resides.
*
* @author $Author: fqian $
* @version $Revision: 1.1 $
*/
public class ClassEnv implements RuntimeConstants
{
int magic;
short version_lo, version_hi;
CP this_class, super_class;
short class_access;
Hashtable cpe, cpe_index;
Vector interfaces;
Vector vars;
Vector methods;
SourceAttr source = null;
Vector generic;
boolean hasSuperClass;
InnerClassAttr inner_class_attr;
boolean classSynth;
SyntheticAttr synthAttr;
DeprecatedAttr deprAttr = null;
SignatureAttr sigAttr = null;
VisibilityAnnotationAttr visAnnotAttr = null;
VisibilityAnnotationAttr invisAnnotAttr = null;
EnclMethAttr encl_meth_attr;
BootstrapMethodsAttribute bsm_attr = null;
public ClassEnv()
{
// Fill in reasonable defaults
magic = JAVA_MAGIC;
version_lo = (short) JAVA1_2_MINOR_VERSION;
version_hi = (short) JAVA1_2_VERSION;
// Initialize bags
cpe = new Hashtable();
cpe_index = null;
interfaces = new Vector();
vars = new Vector();
methods = new Vector();
generic = new Vector();
bsm_attr = null;
}
public void requireJava1_4() {
version_lo = (short) Math.max(version_lo,(short) JAVA1_4_MINOR_HIGH_VERSION);
version_hi = (short) Math.max(version_hi,(short) JAVA1_4_HIGH_VERSION);
}
public void requireJava5() {
version_lo = (short) Math.max(version_lo,(short) JAVA5_MINOR_HIGH_VERSION);
version_hi = (short) Math.max(version_hi,(short) JAVA5_HIGH_VERSION);
}
public void requireJava6() {
version_lo = (short) Math.max(version_lo, (short) JAVA6_MINOR_HIGH_VERSION);
version_hi = (short) Math.max(version_hi, (short) JAVA6_HIGH_VERSION);
}
public void requireJava7() {
version_lo = (short) Math.max(version_lo, (short) JAVA7_MINOR_HIGH_VERSION);
version_hi = (short) Math.max(version_hi, (short) JAVA7_HIGH_VERSION);
}
/**
* Define this class to have this name.
* @param name CPE representing name for class. (This is usually
* a ClassCP)
*/
public void setClass(CP name)
{ this_class = name; addCPItem(name); }
/**
* Define this class to have no superclass. Should only ever
* be used for java.lang.Object
*/
public void setNoSuperClass()
{
hasSuperClass = false;
}
/**
* Define this class to have this superclass
* @param name CPE representing name for class. (This is usually
* a ClassCP)
*/
public void setSuperClass(CP name)
{
hasSuperClass = true;
super_class = name;
addCPItem(name);
}
/**
* Set the class access for this class. Constants understood
* by this are present along with the java Beta distribution.
* @param access number representing access permissions for
* the entire class.
* @see RuntimeConstants
*/
public void setClassAccess(short access)
{ class_access = access; }
/**
* Add this CP to the list of interfaces supposedly implemented by
* this class. Note that the CP ought to be a ClassCP to make
* sense to the VM.
*/
public void addInterface(CP ifc)
{
addCPItem(ifc);
interfaces.addElement(ifc);
}
/**
* Add this to the list of interfaces supposedly implemented
* by this class. Note that each CP is usually a ClassCP.
* @param ilist An array of CP items representing the
* interfaces implemented by this class.
*/
public void addInterface(CP ilist[])
{
for (int i=0; i 32K become negative and then not made wide
/* short */ int getCPIndex(CP cp)
throws jasError
{
if (cpe_index == null) {
throw new jasError("Internal error: CPE index has not been generated");
}
//System.out.println("cp uniq: "+cp.getUniq());
//System.out.println("cpe_index: "+cpe_index);
Integer idx = (Integer)(cpe_index.get(cp.getUniq()));
// LJH -----------------------------
//System.out.println("Getting idx " + idx);
if (idx == null)
throw new jasError("Item " + cp + " not in the class");
return (idx.intValue());
}
public int addBootstrapMethod(MethodHandleCP bsm, CP[] argCPs) {
addCPItem(bsm);
for (CP cp : argCPs) {
addCPItem(cp);
}
if(bsm_attr==null) {
bsm_attr = new BootstrapMethodsAttribute();
bsm_attr.resolve(this);
}
requireJava7();
return bsm_attr.addEntry(bsm, argCPs);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy