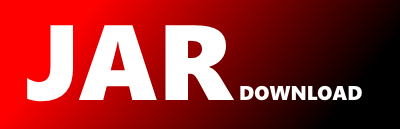
soot.jimple.ParameterRef Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of soot Show documentation
Show all versions of soot Show documentation
A Java Optimization Framework
package soot.jimple;
/*-
* #%L
* Soot - a J*va Optimization Framework
* %%
* Copyright (C) 1997 - 1999 Raja Vallee-Rai
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 2.1 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.Collections;
import java.util.List;
import soot.Type;
import soot.UnitPrinter;
import soot.ValueBox;
import soot.util.Switch;
/**
* ParameterRef
objects are used by Body
objects to refer to the parameter slots on method entry.
*
*
* For instance, in an instance method, the first statement will often be this := @parameter0;
*/
public class ParameterRef implements IdentityRef {
int n;
Type paramType;
/** Constructs a ParameterRef object of the specified type, representing the specified parameter number. */
public ParameterRef(Type paramType, int number) {
this.n = number;
this.paramType = paramType;
}
public boolean equivTo(Object o) {
if (o instanceof ParameterRef) {
return n == ((ParameterRef) o).n && paramType.equals(((ParameterRef) o).paramType);
}
return false;
}
public int equivHashCode() {
return n * 101 + paramType.hashCode() * 17;
}
/** Create a new ParameterRef object with the same paramType and number. */
public Object clone() {
return new ParameterRef(paramType, n);
}
/** Converts the given ParameterRef into a String i.e. @parameter0: .int
. */
public String toString() {
return "@parameter" + n + ": " + paramType;
}
public void toString(UnitPrinter up) {
up.identityRef(this);
}
/** Returns the index of this ParameterRef. */
public int getIndex() {
return n;
}
/** Sets the index of this ParameterRef. */
public void setIndex(int index) {
n = index;
}
@Override
public final List getUseBoxes() {
return Collections.emptyList();
}
/** Returns the type of this ParameterRef. */
public Type getType() {
return paramType;
}
/** Used with RefSwitch. */
public void apply(Switch sw) {
((RefSwitch) sw).caseParameterRef(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy