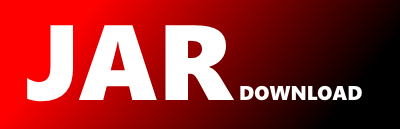
ca.uhn.test.util.AssertJson Maven / Gradle / Ivy
/*-
* #%L
* HAPI FHIR Test Utilities
* %%
* Copyright (C) 2014 - 2024 Smile CDR, Inc.
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
package ca.uhn.test.util;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import jakarta.annotation.Nonnull;
import org.apache.commons.lang3.StringUtils;
import org.assertj.core.api.AbstractAssert;
import org.assertj.core.api.Assertions;
import org.assertj.core.api.SoftAssertions;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import static org.assertj.core.api.Fail.fail;
/**
* Assertj extension to ease testing json strings with few nested quoted strings
*/
public class AssertJson extends AbstractAssert {
public AssertJson(String actual) {
super(actual, AssertJson.class);
}
public static AssertJson assertThat(String actual) {
return new AssertJson(actual);
}
public AssertJson hasPath(String thePath) {
isNotNull();
isNotEmpty(thePath);
Assertions.assertThat(isJsonObjStr(actual)).isTrue();
Map actualMap = getMap(actual);
Assertions.assertThat(actualMap).isNotNull();
getPathMap(thePath);
return this;
}
private AssertJson isNotEmpty(String thePath) {
Assertions.assertThat(thePath).isNotEmpty();
return this;
}
public AssertJson hasKeys(String... theKeys) {
isNotNull();
Map map = getMap(actual);
Assertions.assertThat(map).isNotNull();
Assertions.assertThat(
map.keySet()).containsAll(Arrays.asList(theKeys));
return this;
}
public AssertJson hasExactlyKeys(String... theKeys) {
isNotNull();
Map map = getMap(actual);
Assertions.assertThat(map).isNotNull();
Assertions.assertThat(
map.keySet()).hasSameElementsAs(Arrays.asList(theKeys));
return this;
}
public AssertJson hasExactlyKeysWithValues(List theKeys, List extends Serializable> theValues) {
isNotNull();
if (!checkSizes(theKeys.size(), theValues.size())) {
return this;
}
Map map = getMap(actual);
Assertions.assertThat(map).isNotNull();
Assertions.assertThat(
map.keySet()).hasSameElementsAs(theKeys);
for (int i = 0; i actualMap = getMap(actual);
Assertions.assertThat(actualMap).isNotNull();
Object actualValue = actualMap.get(theKey);
JsonTestTypes actualValueType = getType(actualValue);
JsonTestTypes expectedValueType = getType(theExpectedValue);
if (actualValueType != expectedValueType) {
fail(getDifferentTypesMessage(theKey, actualValueType, expectedValueType));
}
if (isJsonObjStr(theExpectedValue)) {
assertJsonObject(actualMap, theKey, theExpectedValue);
return this;
}
if (isJsonList(theExpectedValue)) {
assertJsonList(actualMap, theKey, theExpectedValue);
return this;
}
Assertions.assertThat(actualMap)
.extracting(theKey)
.isEqualTo(theExpectedValue);
return this;
}
private void assertJsonList(Map theActualMap, String theKey, Object theExpectedValue) {
List> expectedValueList = getList((String) theExpectedValue);
Assertions.assertThat(expectedValueList).isNotNull();
Assertions.assertThat(theActualMap.get(theKey)).isNotNull().isInstanceOf(Collection.class);
List> actualValueList = (List>) theActualMap.get(theKey);
Assertions.assertThat(actualValueList)
.asList()
.hasSameElementsAs(expectedValueList);
}
private JsonTestTypes getType(Object theValue) {
if (theValue instanceof Map, ?>) {
return JsonTestTypes.JSON_OBJECT;
}
if (isJsonObjStr(theValue)) {
getMap((String) theValue);
return JsonTestTypes.JSON_OBJECT;
}
if (isJsonList(theValue)) {
return JsonTestTypes.JSON_LIST;
}
return JsonTestTypes.STRING_NOT_JSON;
}
private String getDifferentTypesMessage(String theKey, JsonTestTypes theActualValueType, JsonTestTypes theExpectedValueType) {
return "Types mismatch. Te expected " + (theKey == null ? " " : "'" + theKey + "' ") +
"value is a " + theExpectedValueType.myDisplay +
" whereas the actual value is a " + theActualValueType.myDisplay;
}
private boolean isJsonList(Object theValue) {
return theValue instanceof Collection> ||
(theValue instanceof String stringValue
&& stringValue.trim().startsWith("[")
&& stringValue.trim().endsWith("]"));
}
private void assertJsonObject(Map theActualMap, String theKey, Object theValue) {
Map expectedValueMap = getMap((String) theValue);
Assertions.assertThat(expectedValueMap).isNotNull();
Assertions.assertThat(theActualMap.get(theKey)).isNotNull().isInstanceOf(Map.class);
@SuppressWarnings("unchecked")
Map actualValueMap = (Map) theActualMap.get(theKey);
SoftAssertions lazyly = new SoftAssertions();
for (String key : actualValueMap.keySet()) {
lazyly.assertThat(actualValueMap)
.as("Unexpected value for key: " + key + ":")
.extracting(key).isEqualTo(expectedValueMap.get(key));
}
lazyly.assertAll();
}
private boolean isJsonObjStr(Object theValue) {
if (theValue instanceof String strValue) {
String trimmed = trimAll(strValue);
return trimmed.startsWith("{") && trimmed.endsWith("}") && isValidJson(trimmed);
}
return false;
}
private String trimAll(String theString) {
return theString.trim().replace("\n", "").replace("\t", "");
}
private boolean isValidJson(String theStrValue) {
getMap(theStrValue);
return true;
}
public AssertJson hasKeysWithValues(List theKeys, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy