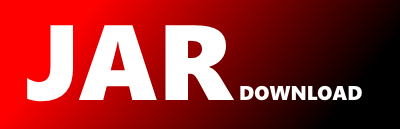
umontreal.iro.lecuyer.charts.QQPlot Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ssj Show documentation
Show all versions of ssj Show documentation
SSJ is a Java library for stochastic simulation, developed under the direction of Pierre L'Ecuyer,
in the Département d'Informatique et de Recherche Opérationnelle (DIRO), at the Université de Montréal.
It provides facilities for generating uniform and nonuniform random variates, computing different
measures related to probability distributions, performing goodness-of-fit tests, applying quasi-Monte
Carlo methods, collecting (elementary) statistics, and programming discrete-event simulations with both
events and processes.
The newest version!
/*
* Class: QQPlot
* Description: qq-plot
* Environment: Java
* Software: SSJ
* Copyright (C) 2001 Pierre L'Ecuyer and Université de Montréal
* Organization: DIRO, Université de Montréal
* @author Richard Simard
* @since May 2011
* SSJ is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License (GPL) as published by the
* Free Software Foundation, either version 3 of the License, or
* any later version.
* SSJ is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
* A copy of the GNU General Public License is available at
GPL licence site.
*/
package umontreal.iro.lecuyer.charts;
import umontreal.iro.lecuyer.probdist.ContinuousDistribution;
import java.util.Arrays;
/**
* This class implements QQ-plot (or quantile-quantile plot)
* objects that compare two probability distributions.
* The data is given as a list of x-coordinates
* (x1, x2,…, xn),
* and one is given a reference continuous probability distribution F(x).
* One first sorts the xi in ascending order, then noted x(i), and
* plots the points
* (F-1(pi), x(i)), where
*
* i = 1, 2,…, n and
* pi = (i - 1/2)/n,
* to see if the data xi comes from the reference distribution F(x).
* The graph of the straight line y = x is also plotted for comparison.
*
*/
public class QQPlot extends XYLineChart {
private double[][] Q; // data points
private double[][] Lin; // line y = x
private void initLinear (double a, double b)
{
// line y = x in [a, b] by steps of h
int m = 100;
double h = (b - a)/ m;
Lin = new double[2][m+1];
for (int i = 0; i <= m; i++)
Lin[0][i] = Lin[1][i] = a + h * i;
}
private void initPoints (ContinuousDistribution dist, double[] data,
int numPoints)
{
int i;
double p;
Q = new double[2][numPoints]; // q_i = cdf^(-1)(p_i)
for (i = 0; i < numPoints; i++)
Q[1][i] = data[i];
Arrays.sort(Q[1]);
for (i = 0; i < numPoints; i++) {
p = (i + 0.5)/numPoints;
Q[0][i] = dist.inverseF(p);
}
}
/**
* Constructs a new QQPlot instance using the points X.
* title is a title, XLabel is a short description of
* the x-axis, and YLabel a short description of the y-axis.
* The plot is a QQ-plot of the points
*
* (F-1(pi), x(i)),
* i = 1, 2,…, n, where
* pi = (i - 1/2)/n,
* xi = X[i-1], x(i) are the sorted points,
* and
* x = F-1(p) = dist.inverseF(p). The points X are not sorted.
*
* @param title chart title.
*
* @param XLabel Label on x-axis.
*
* @param YLabel Label on y-axis.
*
* @param dist Reference distribution
*
* @param X points.
*
*
*/
public QQPlot (String title, String XLabel, String YLabel,
ContinuousDistribution dist, double[] X) {
this (title, XLabel, YLabel, dist, X, X.length);
}
/**
* Similar to the constructor {@link #QQPlot(String,String,String,ContinuousDistribution,double[]) QQPlot}(title, XLabel, YLabel, dist, X) above, except that only the first numPoints of X
* are plotted.
*
* @param title chart title.
*
* @param XLabel Label on x-axis.
*
* @param YLabel Label on y-axis.
*
* @param dist Reference distribution
*
* @param X point set.
*
* @param numPoints number of points to plot
*
*
*/
public QQPlot (String title, String XLabel, String YLabel,
ContinuousDistribution dist, double[] X, int numPoints) {
super();
initPoints (dist, X, numPoints);
initLinear (Q[1][0], Q[1][numPoints-1]);
dataset = new XYListSeriesCollection(Q, Lin);
// --- dashed line for y = x
((XYListSeriesCollection)dataset).setDashPattern(1, "dashed");
init (title, XLabel, YLabel);
}
/**
* Constructs a new QQPlot instance.
* title is a title, XLabel is a short description of
* the x-axis, and YLabel a short description of the y-axis.
* The input vectors in data represents several sets of x-points.
* r determine the set of points to be plotted in the QQ-plot, that is,
* one will plot only the points data[r][i],
* for
* i = 0, 1,…,(n - 1) and a given r, where n is the number
* of points in set r. The points are assumed to follow the distribution
* dist.
*
* @param title chart title.
*
* @param XLabel Label on x-axis.
*
* @param YLabel Label on y-axis.
*
* @param dist Reference distribution
*
* @param data series of point sets.
*
* @param r set of points to plot
*
*/
public QQPlot (String title, String XLabel, String YLabel,
ContinuousDistribution dist, double[][] data, int r) {
this (title, XLabel, YLabel, dist, data[r], data[r].length);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy