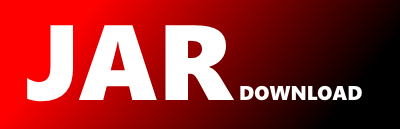
ca.wheatstalk.cdkecskeycloak.KeycloakCluster Maven / Gradle / Ivy
Show all versions of cdk-ecs-keycloak Show documentation
package ca.wheatstalk.cdkecskeycloak;
/**
* A complete Keycloak cluster in a box.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.24.0 (build b722f66)", date = "2021-03-04T07:10:04.621Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = ca.wheatstalk.cdkecskeycloak.$Module.class, fqn = "@wheatstalk/cdk-ecs-keycloak.KeycloakCluster")
public class KeycloakCluster extends software.amazon.awscdk.core.Construct {
protected KeycloakCluster(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected KeycloakCluster(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public KeycloakCluster(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.Nullable ca.wheatstalk.cdkecskeycloak.KeycloakClusterProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), props });
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public KeycloakCluster(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required") });
}
/**
* The ECS service controlling the cluster tasks.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.ecs.BaseService getService() {
return software.amazon.jsii.Kernel.get(this, "service", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.ecs.BaseService.class));
}
/**
* A fluent builder for {@link ca.wheatstalk.cdkecskeycloak.KeycloakCluster}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private ca.wheatstalk.cdkecskeycloak.KeycloakClusterProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
}
/**
* (deprecated) Add the service's WildFly admin console port to a load balancer.
*
* You will
* probably need to use your own Dockerfile to add access to this console.
*
* Default: - not exposed
*
* @return {@code this}
* @deprecated use `adminConsolePortPublisher` instead
* @param adminConsoleListenerProvider Add the service's WildFly admin console port to a load balancer. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder adminConsoleListenerProvider(final ca.wheatstalk.cdkecskeycloak.IListenerInfoProvider adminConsoleListenerProvider) {
this.props().adminConsoleListenerProvider(adminConsoleListenerProvider);
return this;
}
/**
* Add the service's WildFly admin console port to a load balancer.
*
* You will
* probably need to use your own Dockerfile to add access to this console.
*
* Default: - not exposed
*
* @return {@code this}
* @param adminConsolePortPublisher Add the service's WildFly admin console port to a load balancer. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder adminConsolePortPublisher(final ca.wheatstalk.cdkecskeycloak.IPortPublisher adminConsolePortPublisher) {
this.props().adminConsolePortPublisher(adminConsolePortPublisher);
return this;
}
/**
* (experimental) Add capacity provider strategy by CDK escape hatch.
*
* @return {@code this}
* @param capacityProviderStrategy Add capacity provider strategy by CDK escape hatch. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder capacityProviderStrategy(final java.util.List extends software.amazon.awscdk.services.ecs.CfnCluster.CapacityProviderStrategyItemProperty> capacityProviderStrategy) {
this.props().capacityProviderStrategy(capacityProviderStrategy);
return this;
}
/**
* Enable/disable the deployment circuit breaker.
*
* Default: true
*
* @return {@code this}
* @param circuitBreaker Enable/disable the deployment circuit breaker. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder circuitBreaker(final java.lang.Boolean circuitBreaker) {
this.props().circuitBreaker(circuitBreaker);
return this;
}
/**
* CloudMap namespace to use for service discovery.
*
* Default: - creates one named 'keycloak-service-discovery'
*
* @return {@code this}
* @param cloudMapNamespaceProvider CloudMap namespace to use for service discovery. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cloudMapNamespaceProvider(final ca.wheatstalk.cdkecskeycloak.ICloudMapNamespaceInfoProvider cloudMapNamespaceProvider) {
this.props().cloudMapNamespaceProvider(cloudMapNamespaceProvider);
return this;
}
/**
* Fargate task cpu spec.
*
* Default: 1024
*
* @return {@code this}
* @param cpu Fargate task cpu spec. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cpu(final java.lang.Number cpu) {
this.props().cpu(cpu);
return this;
}
/**
* Database server.
*
* Default: - creates a new one
*
* @return {@code this}
* @param databaseProvider Database server. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder databaseProvider(final ca.wheatstalk.cdkecskeycloak.IDatabaseInfoProvider databaseProvider) {
this.props().databaseProvider(databaseProvider);
return this;
}
/**
* How many keycloak cluster members to spin up.
*
* Default: 1
*
* @return {@code this}
* @param desiredCount How many keycloak cluster members to spin up. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder desiredCount(final java.lang.Number desiredCount) {
this.props().desiredCount(desiredCount);
return this;
}
/**
* Provide an ECS cluster.
*
* Default: - a cluster is automatically created.
*
* @return {@code this}
* @param ecsClusterProvider Provide an ECS cluster. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder ecsClusterProvider(final ca.wheatstalk.cdkecskeycloak.IClusterInfoProvider ecsClusterProvider) {
this.props().ecsClusterProvider(ecsClusterProvider);
return this;
}
/**
* Initial grace period for Keycloak to spin up.
*
* Default: 10 minutes
*
* @return {@code this}
* @param healthCheckGracePeriod Initial grace period for Keycloak to spin up. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder healthCheckGracePeriod(final software.amazon.awscdk.core.Duration healthCheckGracePeriod) {
this.props().healthCheckGracePeriod(healthCheckGracePeriod);
return this;
}
/**
* Publish the service's HTTP port.
*
* Default: - a new load balancer is automatically created unless `httpsPort` is given.
*
* @return {@code this}
* @param httpPortPublisher Publish the service's HTTP port. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder httpPortPublisher(final ca.wheatstalk.cdkecskeycloak.IPortPublisher httpPortPublisher) {
this.props().httpPortPublisher(httpPortPublisher);
return this;
}
/**
* (deprecated) Add the service's https port to a load balancer.
*
* When provided, the
* http port is no longer exposed by an http load balancer by default.
*
* Default: - not exposed
*
* @return {@code this}
* @deprecated - use `httpsPortPublisher` instead
* @param httpsListenerProvider Add the service's https port to a load balancer. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder httpsListenerProvider(final ca.wheatstalk.cdkecskeycloak.IListenerInfoProvider httpsListenerProvider) {
this.props().httpsListenerProvider(httpsListenerProvider);
return this;
}
/**
* Publish the service's HTTPS port.
*
* When provided, the http port is no
* longer exposed by default
*
* Default: - not published
*
* @return {@code this}
* @param httpsPortPublisher Publish the service's HTTPS port. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder httpsPortPublisher(final ca.wheatstalk.cdkecskeycloak.IPortPublisher httpsPortPublisher) {
this.props().httpsPortPublisher(httpsPortPublisher);
return this;
}
/**
* Keycloak configuration options.
*
* @return {@code this}
* @param keycloak Keycloak configuration options. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder keycloak(final ca.wheatstalk.cdkecskeycloak.KeycloakContainerExtensionProps keycloak) {
this.props().keycloak(keycloak);
return this;
}
/**
* (deprecated) Add the service's http port to a load balancer.
*
* Default: - a new load balancer is automatically created unless `httpsListenerProvider` is given.
*
* @return {@code this}
* @deprecated use `httpPortPublisher` instead
* @param listenerProvider Add the service's http port to a load balancer. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder listenerProvider(final ca.wheatstalk.cdkecskeycloak.IListenerInfoProvider listenerProvider) {
this.props().listenerProvider(listenerProvider);
return this;
}
/**
* The maximum percentage of healthy tasks during deployments.
*
* @return {@code this}
* @param maxHealthyPercent The maximum percentage of healthy tasks during deployments. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maxHealthyPercent(final java.lang.Number maxHealthyPercent) {
this.props().maxHealthyPercent(maxHealthyPercent);
return this;
}
/**
* Fargate task memory spec.
*
* Default: 2048
*
* @return {@code this}
* @param memoryLimitMiB Fargate task memory spec. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder memoryLimitMiB(final java.lang.Number memoryLimitMiB) {
this.props().memoryLimitMiB(memoryLimitMiB);
return this;
}
/**
* The minimum percentage of healthy tasks during deployments.
*
* @return {@code this}
* @param minHealthyPercent The minimum percentage of healthy tasks during deployments. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder minHealthyPercent(final java.lang.Number minHealthyPercent) {
this.props().minHealthyPercent(minHealthyPercent);
return this;
}
/**
* VPC to use.
*
* Default: - creates one
*
* @return {@code this}
* @param vpcProvider VPC to use. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcProvider(final ca.wheatstalk.cdkecskeycloak.IVpcInfoProvider vpcProvider) {
this.props().vpcProvider(vpcProvider);
return this;
}
/**
* Assign public IPs to the Fargate tasks.
*
* Note: Useful if you don't have a NAT gateway and only public subnets.
*
* Default: false
*
* @return {@code this}
* @param vpcTaskAssignPublicIp Assign public IPs to the Fargate tasks. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcTaskAssignPublicIp(final java.lang.Boolean vpcTaskAssignPublicIp) {
this.props().vpcTaskAssignPublicIp(vpcTaskAssignPublicIp);
return this;
}
/**
* Where to place the instances within the VPC.
*
* Note: Useful when the VPC has no private subnets.
*
* @return {@code this}
* @param vpcTaskSubnets Where to place the instances within the VPC. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcTaskSubnets(final software.amazon.awscdk.services.ec2.SubnetSelection vpcTaskSubnets) {
this.props().vpcTaskSubnets(vpcTaskSubnets);
return this;
}
/**
* @returns a newly built instance of {@link ca.wheatstalk.cdkecskeycloak.KeycloakCluster}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ca.wheatstalk.cdkecskeycloak.KeycloakCluster build() {
return new ca.wheatstalk.cdkecskeycloak.KeycloakCluster(
this.scope,
this.id,
this.props != null ? this.props.build() : null
);
}
private ca.wheatstalk.cdkecskeycloak.KeycloakClusterProps.Builder props() {
if (this.props == null) {
this.props = new ca.wheatstalk.cdkecskeycloak.KeycloakClusterProps.Builder();
}
return this.props;
}
}
}