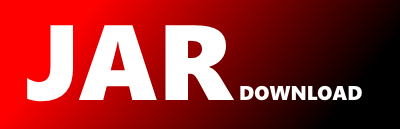
org.directwebremoting.Security Maven / Gradle / Ivy
package org.directwebremoting;
/**
* Some simple replacement utilities to help people protect themselves from
* XSS attacks.
* This class represents some simple filters which may protect from
* simple attacks in low risk environments. There is no replacement for a full
* security review which assesses the risks that you face.
* @author Joe Walker [joe at getahead dot ltd dot uk]
*/
public class Security
{
/**
* Perform the following replacements:
* - & to &
* - < to <
* - > to >
* - ' to '
* - " to "
*
* These replacements are useful when the original sense is important, but
* when we wish to reduce the risk of XSS attacks.
* @param original The string to perform entity replacement on
* @return The original string with &, <, >, ' and " escaped.
* @see #unescapeHtml(String)
*/
public static String escapeHtml(String original)
{
String reply = original;
reply = reply.replace("&", "&");
reply = reply.replace("<", "<");
reply = reply.replace(">", ">");
reply = reply.replace("\'", "'");
reply = reply.replace("\"", """);
return reply;
}
/**
* Perform the following replacements:
* - & to &
* - < to <
* - > to >
* - ' to '
* - " to "
*
* These replacements are useful to reverse the effects of
* {@link #escapeHtml(String)}.
* @param original The string to perform entity replacement on
* @return The original string with &, <, >, ' and " replaced.
* @see #escapeHtml(String)
*/
public static String unescapeHtml(String original)
{
String reply = original;
reply = reply.replace("&", "&");
reply = reply.replace("<", "<");
reply = reply.replace(">", ">");
reply = reply.replace("'", "\'");
reply = reply.replace(""", "\"");
return reply;
}
/**
* Perform the following replacements:
* - & to +
* - < to \\u2039 (‹)
* - > to \\u203A (›)
* - ' to \\u2018 (‘)
* - " to \\u201C (“)
*
* These replacements are useful when readability is more important than
* retaining the exact character string of the original.
* @param original The string to perform entity replacement on
* @return The original string with &, <, >, ' and " escaped.
*/
public static String replaceXmlCharacters(String original)
{
String reply = original;
reply = reply.replace("&", "+");
reply = reply.replace("<", "‹");
reply = reply.replace(">", "›");
reply = reply.replace("'", "‘");
reply = reply.replace("\"", "“");
return reply;
}
/**
* Return true iff the input string contains any of the characters that
* are special to XML: &, <, >, ' or "
* @param original The string to test for XML special characters
* @return True if the characters are found, false otherwise
*/
public static boolean containsXssRiskyCharacters(String original)
{
return (original.indexOf('&') != -1
|| original.indexOf('<') != -1
|| original.indexOf('>') != -1
|| original.indexOf('\'') != -1
|| original.indexOf('\"') != -1);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy