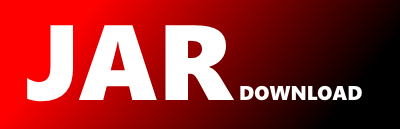
org.directwebremoting.json.parse.impl.ConverterJsonDecoder Maven / Gradle / Ivy
package org.directwebremoting.json.parse.impl;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.directwebremoting.extend.Property;
import org.directwebremoting.json.parse.JsonDecoder;
import org.directwebremoting.json.parse.JsonParseException;
/**
* This class is incomplete
* @author Joe Walker [joe at getahead dot ltd dot uk]
*/
class ConverterJsonDecoder implements JsonDecoder
{
/**
* We need to know what we are decoding into
*/
public ConverterJsonDecoder(Property property)
{
destinations.addLast(property);
}
/* (non-Javadoc)
* @see org.directwebremoting.json.parse.JsonDecoder#getRoot()
*/
public Object getRoot() throws JsonParseException
{
return last;
}
/* (non-Javadoc)
* @see org.directwebremoting.json.parse.impl.StatefulJsonDecoder#beginObject()
*/
public void beginObject(String propertyName) throws JsonParseException
{
//Property property = destinations.getLast();
//Class> type = property.getPropertyType();
stack.addLast(new HashMap());
}
/* (non-Javadoc)
* @see org.directwebremoting.json.parse.impl.StatefulJsonDecoder#endObject()
*/
public void endObject(String propertyName) throws JsonParseException
{
this.last = stack.removeLast();
// Don't try to add the top level object to its parent
if (!stack.isEmpty())
{
add(propertyName, last);
}
}
/* (non-Javadoc)
* @see org.directwebremoting.json.parse.impl.StatefulJsonDecoder#beginArray()
*/
public void beginArray(String propertyName) throws JsonParseException
{
stack.addLast(new ArrayList
© 2015 - 2024 Weber Informatics LLC | Privacy Policy