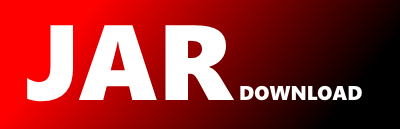
org.directwebremoting.servlet.DownloadHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dwr Show documentation
Show all versions of dwr Show documentation
DWR is easy Ajax for Java. It makes it simple to call Java code directly from Javascript.
It gets rid of almost all the boilerplate code between the web browser and your Java code.
This version 4.0.2 works with Jakarta Servlet 4.0.2.
The newest version!
package org.directwebremoting.servlet;
import java.io.IOException;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
import org.directwebremoting.extend.DownloadManager;
import org.directwebremoting.extend.Handler;
import org.directwebremoting.io.FileTransfer;
import org.directwebremoting.io.OutputStreamLoader;
import org.directwebremoting.util.LocalUtil;
/**
* A DownloadHandler is basically a FileServingServlet that integrates with
* a DownloadManager to purge files from the system that have been downloaded.
* @author Joe Walker [joe at getahead dot ltd dot uk]
*/
public class DownloadHandler implements Handler {
public void handle(HttpServletRequest request, HttpServletResponse response) throws IOException {
String id = request.getPathInfo();
if (!id.startsWith(downloadHandlerUrl)) {
response.sendError(HttpServletResponse.SC_NOT_FOUND);
}
id = id.substring(downloadHandlerUrl.length());
FileTransfer transfer = downloadManager.getFileTransfer(id);
if (transfer == null) {
response.sendError(HttpServletResponse.SC_NOT_FOUND);
} else {
if (transfer.getSize() > 0) {
response.setContentLength((int) transfer.getSize());
}
String defaultType = "attachment";
String type = null;
String suppliedType = request.getParameter("contentDispositionType");
if (suppliedType != null && suppliedType.matches("^[-A-Za-z]+$")) {
type = suppliedType;
}
String filename = transfer.getFilename();
if (type != null || filename != null) {
response.setHeader("Content-disposition",
(type != null ? type : defaultType)
+ (filename != null ? "; filename=\"" + filename + "\"" : ""));
}
response.setContentType(transfer.getMimeType());
OutputStreamLoader loader = null;
try {
loader = transfer.getOutputStreamLoader();
loader.load(response.getOutputStream());
} finally {
LocalUtil.close(loader);
}
}
}
/**
* @param downloadManager The new DownloadManager
*/
public void setDownloadManager(DownloadManager downloadManager)
{
this.downloadManager = downloadManager;
}
/**
* The URL part which we attach to the downloads.
* @param downloadHandlerUrl The URL for this Handler.
*/
public void setDownloadHandlerUrl(String downloadHandlerUrl)
{
this.downloadHandlerUrl = downloadHandlerUrl;
}
/**
* The place we store files for later download
*/
private DownloadManager downloadManager;
/**
* The URL part which we attach to the downloads.
*/
protected String downloadHandlerUrl;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy