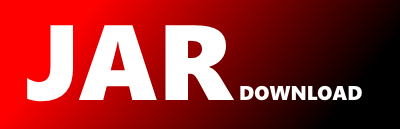
cat.inspiracio.html.HTMLDOMImplementation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of html-parser Show documentation
Show all versions of html-parser Show documentation
HTML-parser provides a parser for HTML 5 that produces
HTML 5 document object model.
It aims to be a Java-implementation of
http://www.w3.org/TR/html5/.
It is for use in the server. It does not implement features
that are relevant in the client, like event handling.
It is for use from javascript, via Java's scripting library.
The newest version!
/*
Copyright 2015 Alexander Bunkenburg
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package cat.inspiracio.html;
import org.w3c.dom.DOMException;
import org.w3c.dom.DocumentType;
import cat.inspiracio.dom.InitialDOMImplementation;
/** Builds html5 documents.
*
* Public visibility so that it can be extended.
* */
public class HTMLDOMImplementation
extends InitialDOMImplementation
implements org.w3c.dom.html.HTMLDOMImplementation //maybe don't need this
{
/** Knows how to instantiate elements for each tag. */
private ElementCreator creator=new ElementCreator();
/** protected so that outside of this package, only subclasses can instantiate. */
protected HTMLDOMImplementation(){super();}
/** XXX Investigate the features and return the right value.
* XML 2.0: false
* */
@Override public boolean hasFeature(String feature, String version){
//System.out.println("hasFeature(" + feature + ", " + version + ")");
return false;
}
/** Called for newDocument() */
@Override public HTMLDocument createDocument(String namespaceURI, String qualifiedName, DocumentType doctype)throws DOMException{
String title=null;
return createHTMLDocument(title);
}
/** Creates an empty HTMLDocument
.
*
* If the title is not null, already creates the elements html/head/title.
*
* @param title The title of the document to be set as the content of the
* title
element, through a child Text
node.
*
* @return A new HTMLDocument
object.
*/
@Override public HTMLDocument createHTMLDocument(String title) {
HTMLDocumentImp d=new HTMLDocumentImp(this);
if(title!=null)
d.setTitle(title);
return d;
}
/** Register a class as implementation for a custom element.
*
* @param cl A class for the custom element.
* The class must have a public constructor with one parameter of type HTMLDocumentImp
* that calls the super-constructor with two parameters: the same HTMLDocumentImp and the desired tag name.
*
* @param tags Registers the class for these tag names.
*
* There may be zero tag names. In that case, the implementation will try to get the tag name
* from the class by instantiating it (with owner == null) and calling getTagName().
*
* There may be exactly one tag name. That is a usual case.
*
* There may be several tag names. In that case, the constructor of the class must remember the
* tag name.
*
* */
public void register(Class extends HTMLElementImp> cl, String... tags){creator.register(cl, tags);}
/** Creates an element.
* @param d
* @param tag
* */
HTMLElement createElement(HTMLDocumentImp d, String tag){return creator.create(d, tag);}
/** Create an element of this class.
*
* Convenience during java programming: the return type is exact.
*
* Not implemented for all element classes.
*
* Some element classes are used to several tags,
* for those this method does not work.
*
* @param A subinterface of HTMLElement that represents one html element
* @param c The class object
* @return A fresh instance of the element
* @throws UnsupportedOperationException Not (yet) implemented for this interface.
* */
T createElement(HTMLDocumentImp d, Class c){return creator.create(d, c);}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy