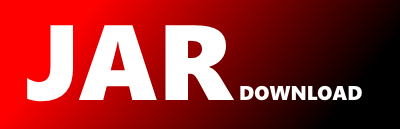
cat.inspiracio.html.HTMLFormElementImp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of html-parser Show documentation
Show all versions of html-parser Show documentation
HTML-parser provides a parser for HTML 5 that produces
HTML 5 document object model.
It aims to be a Java-implementation of
http://www.w3.org/TR/html5/.
It is for use in the server. It does not implement features
that are relevant in the client, like event handling.
It is for use from javascript, via Java's scripting library.
The newest version!
/*
Copyright 2015 Alexander Bunkenburg
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package cat.inspiracio.html;
import org.w3c.dom.NodeList;
class HTMLFormElementImp extends HTMLElementImp implements HTMLFormElement {
private static final long serialVersionUID = 11269356676270397L;
HTMLFormElementImp(HTMLDocumentImp owner){super(owner, "form");}
@Override public HTMLFormElementImp cloneNode(boolean deep){
return (HTMLFormElementImp)super.cloneNode(deep);
}
// methods ----------------------------------------------
@Override public String getAcceptCharset(){return getAttribute("accept-charset");}
@Override public void setAcceptCharset(String accept){setAttribute("accept-charset", accept);}
@Override public String getAction(){
if(hasAttribute("action"))return getAttribute("action");
HTMLDocument document=getOwnerDocument();
return document.getDocumentURI();
}
@Override public void setAction(String action){setAttribute("action", action);}
@Override public String getAutocomplete(){return getAttribute("autocomplete");}
@Override public void setAutocomplete(String auto){setAttribute("autocomplete", auto);}
@Override public String getEnctype(){
if(!hasAttribute("enctype"))
return "";//There is no missing value default for the enctype attribute.
String s=getAttribute("enctype");
boolean valid=EncType.isValid(s);
if(valid)return s;
return EncType.getInvalidDefault().toString();
}
@Override public void setEnctype(String enc){
boolean valid=EncType.isValid(enc);
if(!valid)enc=EncType.getInvalidDefault().toString();
setAttribute("enctype", enc);
}
@Override public String getEncoding(){return getEnctype();}
@Override public void setEncoding(String s){setEnctype(s);}
@Override public String getMethod(){return getAttribute("method");}
@Override public void setMethod(String s){setAttribute("method", s);}
@Override public boolean getNoValidate(){return getAttributeBoolean("novalidate");}
@Override public void setNoValidate(boolean b){setAttribute("novalidate", b);}
@Override public int getLength(){
HTMLFormControlsCollection controls=getElements();
if(controls==null)return 0;
return controls.getLength();
}
@Override public String getTarget(){return getAttribute("target");}
@Override public void setTarget(String s){setAttribute("target", s);}
/** Returns an HTMLCollection of the form controls in the form (excluding image buttons for historical reasons).
*
* The elements IDL attribute must return an HTMLFormControlsCollection
* rooted at the Document node while the form element is in a Document and
* rooted at the form element itself when it is not,
* whose filter matches listed elements whose form owner is the form element,
* with the exception of input elements whose type attribute is in the Image Button state,
* which must, for historical reasons, be excluded from this particular collection. */
@Override public HTMLFormControlsCollection getElements(){return new HTMLFormControlsCollectionImp(this);}
/** http://www.w3.org/TR/html5/forms.html#dom-form-item */
@Override public HTMLElement item(int index){
HTMLFormControlsCollection elements=getElements();
if(elements==null)return null;
return elements.item(index);
}
/** http://www.w3.org/TR/html5/forms.html#dom-form-nameditem
*
* Returns the form control (or, if there are several, a RadioNodeList of the
* form controls) in the form with the given ID or name (excluding image
* buttons for historical reasons); or, if there are none, returns the img
* element with the given ID.
*
* Once an element has been referenced using a particular name, that name
* will continue being available as a way to reference that element in this
* method, even if the element's actual ID or name changes, for as long as
* the element remains in the Document.
*
* If there are multiple matching items, then a RadioNodeList object containing
* all those elements is returned.
* */
@Override public Object namedItem(String name){
if(name==null || 0==name.length())return null;
//1. Let candidates be a live RadioNodeList object containing all the listed
//elements whose form owner is the form element that have either an id attribute
//or a name attribute equal to name, with the exception of input elements whose
//type attribute is in the Image Button state, in tree order.
RadioNodeListImp candidates=new RadioNodeListImp();
HTMLFormControlsCollection controls=getElements();
if(controls!=null)
for(ListedElement e : controls){
if(name.equals(e.getId()) || name.equals(e.getName()))
candidates.add(e);
}
//2. If candidates is empty, let candidates be a live RadioNodeList object
//containing all the img elements that are descendants of the form element
//and that have either an id attribute or a name attribute equal to name, in tree order.
if(candidates.getLength()==0){
NodeList images=getElementsByTagName("img");
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy