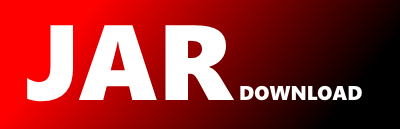
cat.inspiracio.html.HTMLInputElementImp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of html-parser Show documentation
Show all versions of html-parser Show documentation
HTML-parser provides a parser for HTML 5 that produces
HTML 5 document object model.
It aims to be a Java-implementation of
http://www.w3.org/TR/html5/.
It is for use in the server. It does not implement features
that are relevant in the client, like event handling.
It is for use from javascript, via Java's scripting library.
The newest version!
/*
Copyright 2015 Alexander Bunkenburg
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package cat.inspiracio.html;
import static java.lang.Double.NaN;
import static java.lang.Double.isInfinite;
import static java.lang.Double.isNaN;
import static java.lang.Math.ceil;
import static java.lang.Math.floor;
import static java.lang.Math.round;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Date;
import java.util.List;
import java.util.TimeZone;
import org.w3c.dom.Element;
import cat.inspiracio.dom.InvalidStateError;
import cat.inspiracio.file.File;
import cat.inspiracio.script.TypeError;
class HTMLInputElementImp extends LabelableElementImp implements HTMLInputElement {
private static final long serialVersionUID = -1758402136570933694L;
private static final String DATE_PATTERN="yyyy-MM-dd";
HTMLInputElementImp(HTMLDocumentImp owner){super(owner, "input");}
@Override public HTMLInputElementImp cloneNode(boolean deep){
return (HTMLInputElementImp)super.cloneNode(deep);
}
// methods ----------------------------------------------
@Override public String getAccept(){return getAttribute("accept");}
@Override public void setAccept(String accept){setAttribute("accept", accept);}
@Override public String getAlt(){return getAttribute("alt");}
@Override public void setAlt(String alt){setAttribute("alt", alt);}
@Override public String getAutocomplete(){return getAttribute("autocomplete");}
@Override public void setAutocomplete(String s){setAttribute("autocomplete", s);}
@Override public boolean getAutofocus(){return getAttributeBoolean("autofocus");}
@Override public void setAutofocus(boolean auto){setAttribute("autofocus", auto);}
@Override public boolean getDefaultChecked(){return getAttributeBoolean("checked");}
@Override public void setDefaultChecked(boolean defaultChecked){setAttribute("checked", defaultChecked);}
@Override public boolean getChecked(){return getAttributeBoolean("checked");}
@Override public void setChecked(boolean checked){setAttribute("checked", checked);}
@Override public String getDirName(){return getAttribute("dirname");}
@Override public void setDirName(String name){setAttribute("dirname", name);}
@Override public boolean getDisabled(){return getAttributeBoolean("disabled");}
@Override public void setDisabled(boolean disabled){setAttribute("disabled", disabled);}
/** First checks explicit form-attribute for a form-id, then looks for an enclosing form. */
@Override public HTMLFormElement getForm(){
if(hasAttribute("form")){
String id=getAttribute("form");
return (HTMLFormElement)getElementById(id);
}
return super.getForm();
}
/** Returns just an empty list. */
@Override public List getFiles(){return new ArrayList();}
@Override public String getFormAction(){
String action=getAttribute("formaction");
if(action==null || 0==action.length()){
//return document's address
HTMLDocument d=getOwnerDocument();
return d.getURL();
}
return action;
}
@Override public void setFormAction(String action){setAttribute("formaction", action);}
@Override public String getFormEncType(){
if(!hasAttribute("formenctype"))
return "";//There is no missing value default for the formenctype attribute.
String s=getAttribute("formenctype");
boolean valid=EncType.isValid(s);
if(valid)return s;
return EncType.getInvalidDefault().toString();
}
@Override public void setFormEncType(String enc){
boolean valid=EncType.isValid(enc);
if(!valid)enc=EncType.getInvalidDefault().toString();
setAttribute("formenctype", enc);
}
@Override public String getFormMethod(){return getAttribute("formmethod");}
@Override public void setFormMethod(String method){setAttribute("formmethod", method);}
@Override public boolean getFormNoValidate(){return getAttributeBoolean("formnovalidate");}
@Override public void setFormNoValidate(boolean b){setAttribute("formnovalidate", b);}
@Override public String getFormTarget(){return getAttribute("formtarget");}
@Override public void setFormTarget(String target){setAttribute("formtarget", target);}
@Override public int getHeight(){return getAttributeInt("height");}
@Override public void setHeight(int height){setAttribute("height", height);}
@Override public boolean getIndeterminate(){return getAttributeBoolean("indeterminate");}
@Override public void setIndeterminate(boolean in){setAttribute("indeterminate", in);}
/** The list IDL attribute must return the current suggestions source element,
* if any, or null otherwise.
*
* The suggestions source element is the first element in the document in tree order
* to have an ID equal to the value of the list attribute, if that element is a
* datalist element. If there is no list attribute, or if there is no element with
* that ID, or if the first element with that ID is not a datalist element, then
* there is no suggestions source element.
*
* Alex says: this API is not so good, because I would expect setList(s) and getList()
* for the attribute, and getDataList() for the HTML element. */
@Override public HTMLElement getList(){
String list=getAttribute("list");
if(list==null || 0==list.length())return null;
HTMLDocument d=getOwnerDocument();
Element e=d.getElementById(list);//should be in tree order
if(e==null)return null;
if(e instanceof HTMLDataListElement)
return (HTMLDataListElement)e;
return null;
}
@Override public String getMax(){return getAttribute("max");}
@Override public void setMax(String max){setAttribute("max", max);}
@Override public int getMaxLength(){return getAttributeInt("maxlength");}
@Override public void setMaxLength(int maxLength){setAttribute("maxlength", maxLength);}
@Override public String getMin(){return getAttribute("min");}
@Override public void setMin(String min){setAttribute("min", min);}
@Override public int getMinLength(){return getAttributeInt("minlength");}
@Override public void setMinLength(int length){setAttribute("minlength", length);}
@Override public boolean getMultiple(){return getAttributeBoolean("multiple");}
@Override public void setMultiple(boolean multiple){setAttribute("multiple", multiple);}
@Override public String getName(){return getAttribute("name");}
@Override public void setName(String name){setAttribute("name", name);}
@Override public String getPattern(){return getAttribute("pattern");}
@Override public void setPattern(String pattern){setAttribute("pattern", pattern);}
@Override public String getPlaceHolder(){return getAttribute("placeholder");}
@Override public void setPlaceHolder(String holder){setAttribute("placeholder", holder);}
@Override public boolean getReadOnly(){return getAttributeBoolean("readonly");}
@Override public void setReadOnly(boolean only){setAttribute("readonly", only);}
@Override public boolean getRequired(){return getAttributeBoolean("required");}
@Override public void setRequired(boolean required){setAttribute("required", required);}
@Override public int getSize(){return getAttributeInt("size");}
@Override public void setSize(int size){setAttribute("size", size);}
@Override public String getSrc(){return getAttribute("src");}
@Override public void setSrc(String src){setAttribute("src", src);}
@Override public String getStep(){return getAttribute("step");}
@Override public void setStep(String step){setAttribute("step", step);}
/** Should return "" if type is not one of the enumerated values. */
@Override public String getType(){return getAttribute("type");}
@Override public void setType(String type){
//Could check whether the argument really is a type.
//At least put in lower case.
if(null!=type)
type=type.toLowerCase();
setAttribute("type", type);
}
@SuppressWarnings("unused")
private boolean isButton(){return "button".equals(getType());}
@SuppressWarnings("unused")
private boolean isCheckBox(){return "checkbox".equals(getType());}
@SuppressWarnings("unused")
private boolean isColor(){return "color".equals(getType());}
private boolean isDate(){return "date".equals(getType());}
@SuppressWarnings("unused")
private boolean isEmail(){return "email".equals(getType());}
@SuppressWarnings("unused")
private boolean isFile(){return "file".equals(getType());}
@SuppressWarnings("unused")
private boolean isHidden(){return "hidden".equals(getType());}
@SuppressWarnings("unused")
private boolean isImage(){return "image".equals(getType());}
private boolean isNumber(){return "number".equals(getType());}
@SuppressWarnings("unused")
private boolean isPassword(){return "password".equals(getType());}
@SuppressWarnings("unused")
private boolean isRadio(){return "radio".equals(getType());}
private boolean isRange(){return "range".equals(getType());}
@SuppressWarnings("unused")
private boolean isReset(){return "reset".equals(getType());}
@SuppressWarnings("unused")
private boolean isSearch(){return "search".equals(getType());}
@SuppressWarnings("unused")
private boolean isSubmit(){return "submit".equals(getType());}
@SuppressWarnings("unused")
private boolean isTel(){return "tel".equals(getType());}
@SuppressWarnings("unused")
private boolean isText(){return "text".equals(getType());}
private boolean isTime(){return "time".equals(getType());}
@SuppressWarnings("unused")
private boolean isURL(){return "url".equals(getType());}
@Override public String getDefaultValue(){return getAttribute("value");}
@Override public void setDefaultValue(String v){setAttribute("value", v);}
@Override public String getValue(){return getAttribute("value");}
/** Setting value to null or "" is removing it. */
@Override public void setValue(String v){
if(v==null || 0==v.length())
removeAttribute("value");
else
setAttribute("value", v);
}
/** http://www.w3.org/TR/html5/forms.html#dom-input-valueasdate */
@Override public Date getValueAsDate(){
if(!isDate() && !isTime())return null;
String value=getValue();
if(isDate())
return parseDate(value);
if(isTime())
return parseTime(value);
return null;
}
@Override public void setValueAsDate(Date d){
if(!isDate() && !isTime())
throw new InvalidStateError();
if(isDate()){
String s=formatDate(d);
setAttribute("value", s);
return;
}
if(isTime()){
String s=formatTime(d);
setAttribute("value", s);
return;
}
}
/** http://www.w3.org/TR/html5/forms.html#dom-input-valueasnumber
* Gets the value as double, according to type, or NaN. */
@Override public double getValueAsNumber(){
if(!isDate() && !isTime() && !isNumber() && !isRange())
return NaN;
if(isDate() || isTime()){
Date d=getValueAsDate();
if(d==null)
return NaN;
return d.getTime();
}
return getAttribute("value", NaN);
}
@Override public void setValueAsNumber(double v){
if(isInfinite(v))
throw new TypeError();
if(!isDate() && !isTime() && !isNumber() && !isRange())
throw new InvalidStateError();
if(isNaN(v)){
setValue("");
return;
}
if(isDate() || isTime()){
long l=round(v);
Date d=new Date(l);
setValueAsDate(d);
return;
}
setAttribute("value", v);
}
@Override public int getWidth(){return getAttributeInt("width");}
@Override public void setWidth(int width){setAttribute("width", width);}
// Stepping -----------------------------------------------------------------------
/** Applies to types "date", "time", "number", "range" */
/** http://www.w3.org/TR/html5/forms.html#dom-input-stepup */
@Override public void stepDown(){step(-1);}//-1 steps upwards
@Override public void stepDown(int n){step(-n);}//-n steps upwards
@Override public void stepUp(){step(1);}
@Override public void stepUp(int n){step(n);}
/** Stepping up or down.
*
* @param n How many upwards steps?
* @throws InvalidStateError
* The step() function does not apply to this type, or
* there is no allowed value step. */
private void step(int n)throws InvalidStateError{
if(n==0)return;
boolean down=n<0;
//Does step apply to this type?
if(!stepApplies())throw new InvalidStateError();
//Have we got a step?
double step=step();//throws InvalidStateError
//Check min and max. Must have min < max.
//3. If the element has a minimum and a maximum and the minimum is greater
//than the maximum, then abort these steps.
double min=min();
double max=max();
if(isNumber(min) && isNumber(max)){
if(max
© 2015 - 2025 Weber Informatics LLC | Privacy Policy