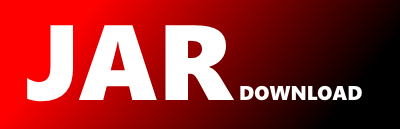
cat.inspiracio.html.HTMLOptionsCollection Maven / Gradle / Ivy
/*
Copyright 2015 Alexander Bunkenburg
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package cat.inspiracio.html;
import cat.inspiracio.dom.HTMLCollection;
/** Spec */
public interface HTMLOptionsCollection extends HTMLCollection, Iterable{
/** Returns the item with index index from the collection.
* The items are sorted in tree order. */
@Override HTMLOptionElement item(int index);
/** Returns the number of elements in the collection. */
@Override int getLength();
/** Sets the number of elements in the collection.
* When set to a smaller number, truncates the number of option elements
* in the corresponding container. When set to a greater number, adds
* new blank option elements to that container.
* @param length the new length */
void setLength(int length);
/** Sets an option element.
*
* May increase the length and thereby create more option elements.
*
* setter creator void (unsigned long index, HTMLOptionElement? option);
*
* @param index the index
* @param option If null, deletes the option at index. */
void set(int index, HTMLOptionElement option);
/** Returns the item with ID or name name from the collection.
* If there are multiple matching items, then the first is returned.
*
* Same as get(name). */
@Override HTMLOptionElement namedItem(String name);
/** Returns the item with ID or name name from the collection.
* If there are multiple matching items, then the first is returned.
*
* Same as namedItem(name).
* @param name the name
* @return the element */
HTMLOptionElement get(String name);
/** Inserts element at the end of the list.
*
* This method will throw a HierarchyRequestError exception if element
* is an ancestor of the element into which it is to be inserted.
* @param option the new option */
void add(HTMLOptionElement option);
/** Inserts element before the node given by before.
*
* This method will throw a HierarchyRequestError exception if element
* is an ancestor of the element into which it is to be inserted.
* @param option the new option
* @param before insert it before this one */
void add(HTMLOptionElement option, HTMLElement before);
/** Inserts element before the node given by before.
*
* The before argument can be a number, in which case element is inserted
* before the item with that number.
*
* If before is a number out of range, then element
* will be added at the end of the list.
*
* This method will throw a HierarchyRequestError exception if element
* is an ancestor of the element into which it is to be inserted.
*
* @param option the new option
* @param before insert it before this one */
void add(HTMLOptionElement option, int before);
/** Inserts element at the end of the list.
*
* This method will throw a HierarchyRequestError exception if element
* is an ancestor of the element into which it is to be inserted.
*
* @param group the new group */
void add(HTMLOptGroupElement group);
/** Inserts element before the node given by before.
*
* If before is null, then element
* will be added at the end of the list.
*
* This method will throw a HierarchyRequestError exception if element
* is an ancestor of the element into which it is to be inserted.
*
* @param group the new group
* @param before insert before this element */
void add(HTMLOptGroupElement group, HTMLElement before);
/** Inserts element before the node given by before.
*
* The before argument can be a number, in which case element is inserted
* before the item with that number.
*
* If before is a number out of range, then element
* will be added at the end of the list.
*
* This method will throw a HierarchyRequestError exception if element
* is an ancestor of the element into which it is to be inserted.
*
* @param group the new group
* @param before insert before this element */
void add(HTMLOptGroupElement group, int before);
/** Removes an option from the group.
* spec
*
* @param index If there is no option with this index, does nothing.
* */
void remove(int index);
int getSelectedIndex();
void setSelectedIndex(int index);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy