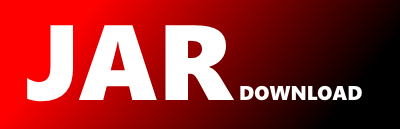
cat.inspiracio.html.HTMLOptionsCollectionImp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of html-parser Show documentation
Show all versions of html-parser Show documentation
HTML-parser provides a parser for HTML 5 that produces
HTML 5 document object model.
It aims to be a Java-implementation of
http://www.w3.org/TR/html5/.
It is for use in the server. It does not implement features
that are relevant in the client, like event handling.
It is for use from javascript, via Java's scripting library.
The newest version!
/*
Copyright 2015 Alexander Bunkenburg
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package cat.inspiracio.html;
import java.util.Iterator;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import cat.inspiracio.dom.HierarchyRequestError;
import cat.inspiracio.dom.NotFoundError;
/** A collection view of the option elements in a select element.
* The collection is live: changing the collection changes the
* children of the select element. */
class HTMLOptionsCollectionImp implements HTMLOptionsCollection {
private static final long serialVersionUID = 3214081886714905353L;
// state ------------------------------------------
private HTMLSelectElement select;
// construction -----------------------------------
HTMLOptionsCollectionImp(HTMLSelectElement s){select=s;}
// methods -----------------------------------------
@Override public Iterator iterator(){
final NodeList options=getOptions();
return new Iterator(){
/** The next to be delivered */
int index=0;
@Override public boolean hasNext(){return indexSpec
* */
@Override public void set(int index, HTMLOptionElement option){
if(option==null){
remove(index);
return;
}
NodeList options=getOptions();
int length=options.getLength();
int n=index-length;
if(0Spec */
@Override public void add(HTMLOptionElement option){add(option, null);}
/** Tests whether an element is an ancestor of another.
*
* Equals: false. (This is probably another special case for the client.)
*
* @param ancestor If null, return false.
* @param descendant If null, return false.
*
* @return Whether ancestor really is an ancestor of descendant.
* */
private boolean isAncestor(HTMLElement ancestor, Node descendant){
if(ancestor==null)return false;
if(descendant==null)return false;
if(ancestor==descendant)return false;
Node parent=descendant.getParentNode();
while(parent!=null){
if(parent==ancestor)return true;
parent=parent.getParentNode();
}
return false;
}
@Override public void add(HTMLOptionElement option, HTMLElement before){_add(option, before);}
private void _add(HTMLElement element, Node before){
if(element==null)return;
//1. If element is an ancestor of the select,
//then throw a HierarchyRequestError exception and abort these steps.
if(isAncestor(element, select))
throw new HierarchyRequestError();
//2. If before is an element, but that element isn't a descendant of the
//select element on which the HTMLOptionsCollection is rooted, then
//throw a NotFoundError exception and abort these steps.
if(before!=null && !isAncestor(select, before))
throw new NotFoundError();
//3. If element and before are the same element, then return and abort
//these steps.
if(element==before)return;
//4. If before is a node, then let reference be that node. Otherwise,
//if before is an integer, and there is a before'th node in the
//collection, let reference be that node. Otherwise, let reference be
//null.
Node reference=before;
//5. If reference is not null, let parent be the parent node of
//reference. Otherwise, let parent be the select element on which the
//HTMLOptionsCollection is rooted.
Node parent= reference!=null ? reference.getParentNode() : select;
//6. Act as if the DOM insertBefore() method was invoked on the parent
//node, with element as the first argument and reference as the second
//argument.
parent.insertBefore(element, reference);
}
@Override public void add(HTMLOptionElement option, int before){_add(option, before);}
private void _add(HTMLElement element, int before){
//4. If before is a node, then let reference be that node. Otherwise,
//if before is an integer, and there is a before'th node in the
//collection, let reference be that node. Otherwise, let reference be
//null.
NodeList options=getOptions();
Node reference=options.item(before);
_add(element, reference);
}
@Override public void add(HTMLOptGroupElement group){add(group, null);}
@Override public void add(HTMLOptGroupElement group, HTMLElement before){_add(group, before);}
@Override public void add(HTMLOptGroupElement group, int before){_add(group, before);}
/** Deletes the option at index. */
@Override public void remove(int index){
NodeList options=getOptions();
Node option=options.item(index);//may be null
if(option==null)return;
Node parent=option.getParentNode();
parent.removeChild(option);
}
@Override public int getSelectedIndex(){
for(int i=0 ; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy