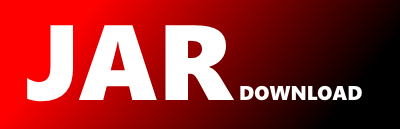
cat.inspiracio.html.HTMLTextAreaElementImp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of html-parser Show documentation
Show all versions of html-parser Show documentation
HTML-parser provides a parser for HTML 5 that produces
HTML 5 document object model.
It aims to be a Java-implementation of
http://www.w3.org/TR/html5/.
It is for use in the server. It does not implement features
that are relevant in the client, like event handling.
It is for use from javascript, via Java's scripting library.
The newest version!
/*
Copyright 2015 Alexander Bunkenburg
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package cat.inspiracio.html;
class HTMLTextAreaElementImp extends LabelableElementImp implements HTMLTextAreaElement {
private static final long serialVersionUID = -6769835618085880755L;
HTMLTextAreaElementImp(HTMLDocumentImp owner){super(owner, "textarea");}
@Override public HTMLTextAreaElementImp cloneNode(boolean deep){
return (HTMLTextAreaElementImp)super.cloneNode(deep);
}
// methods ----------------------------------------------
@Override public String getAutocomplete(){return getAttribute("autocomplete");}
@Override public void setAutocomplete(String s){setAttribute("autocomplete", s);}
@Override public boolean getAutofocus(){return getAttributeBoolean("autofocue");}
@Override public void setAutofocus(boolean b){setAttribute("autofocus", b);}
@Override public int getCols(){return getAttribute("cols", 20);}
@Override public void setCols(int cols){setAttribute("cols", cols);}
@Override public String getDirName(){return getAttribute("dirname");}
@Override public void setDirName(String dir){setAttribute("dirname", dir);}
@Override public boolean getDisabled(){return getAttributeBoolean("disabled");}
@Override public void setDisabled(boolean b){setAttribute("disabled", b);}
/** First checks explicit form-attribute for a form-id, then looks for an enclosing form. */
@Override public HTMLFormElement getForm(){
if(hasAttribute("form")){
String id=getAttribute("form");
return (HTMLFormElement) getElementById(id);
}
return super.getForm();
}
@Override public int getMaxLength(){return getAttributeInt("maxlength");}
@Override public void setMaxLength(int max){setAttribute("maxlength", max);}
@Override public int getMinLength(){return getAttributeInt("minlength");}
@Override public void setMinLength(int min){setAttribute("minlength", min);}
@Override public String getName(){return getAttribute("name");}
@Override public void setName(String name){setAttribute("name", name);}
@Override public String getPlaceholder(){return getAttribute("placeholder");}
@Override public void setPlaceholder(String s){setAttribute("placeholder", s);}
@Override public boolean getReadOnly(){return getAttributeBoolean("readonly");}
@Override public void setReadOnly(boolean b){setAttribute("readonly", b);}
@Override public boolean getRequired(){return getAttributeBoolean("required");}
@Override public void setRequired(boolean b){setAttribute("required", b);}
@Override public int getRows(){return getAttribute("rows", 2);}
@Override public void setRows(int rows){setAttribute("rows", rows);}
@Override public String getWrap(){return getAttribute("wrap", "soft");}
@Override public void setWrap(String wrap){setAttribute("wrap", wrap);}
@Override public String getType(){return "textarea";}
@Override public String getDefaultValue(){return getTextContent();}
@Override public void setDefaultValue(String value){setTextContent(value);}
/** Gets the text content. No line-ending transformations. */
@Override public String getValue(){return getTextContent();}
/** Sets the text content. No line-ending transformations. */
@Override public void setValue(String value){setTextContent(value);}
@Override public int getTextLength(){
String text=getValue();
if(text!=null)
return text.length();
return 0;
}
// validation: not implemented ------------------------------------------
@Override public boolean getWillValidate(){throw new UnsupportedOperationException();}
@Override public ValidityState getValidity(){throw new UnsupportedOperationException();}
@Override public String getValidationMessage(){throw new UnsupportedOperationException();}
@Override public boolean checkValidity(){throw new UnsupportedOperationException();}
@Override public void setCustomValidity(String error){throw new UnsupportedOperationException();}
// selection: not implemented --------------------------------------------
@Override public void select(){throw new UnsupportedOperationException();}
@Override public int getSelectionStart(){throw new UnsupportedOperationException();}
@Override public void setSelectionStart(int start){throw new UnsupportedOperationException();}
@Override public int getSelectionEnd(){throw new UnsupportedOperationException();}
@Override public void setSelectionEnd(int end){throw new UnsupportedOperationException();}
@Override public void setRangeText(String replacement){throw new UnsupportedOperationException();}
@Override public void setRangeText(String replacement, int start, int end){throw new UnsupportedOperationException();}
@Override public void setRangeText(String replacement, int start, int end, SelectionMode mode){throw new UnsupportedOperationException();}
@Override public void setSelectionRange(int start, int end){throw new UnsupportedOperationException();}
@Override public void setSelectionRange(int start, int end, String direction){throw new UnsupportedOperationException();}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy