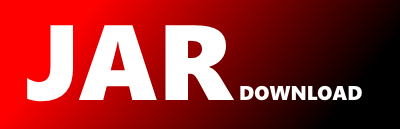
cat.inspiracio.servlet.jsp.InitialPageContext Maven / Gradle / Ivy
/*
Copyright 2015 Alexander Bunkenburg
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package cat.inspiracio.servlet.jsp;
import java.io.IOException;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import javax.servlet.Servlet;
import javax.servlet.ServletConfig;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import javax.servlet.jsp.JspWriter;
import javax.servlet.jsp.PageContext;
import javax.servlet.jsp.el.ExpressionEvaluator;
import javax.servlet.jsp.el.VariableResolver;
/** Superclass for PageContext implementations.
* This class gives the convenience methods and a basis for page scope.
* The rest of the methods throws RuntimeException("not implemented").
* @author alex
* */
class InitialPageContext extends PageContext {
// state --------------------------------------------------
/** If this is a request for an exception page, here the exception. */
private Exception exception;
/** Basis for a page scope implementation. */
private Map page=new HashMap();
private HttpServlet servlet;//page
private JspWriter out;
private HttpServletRequest request;
private HttpServletResponse response;
private HttpSession session;
private ServletConfig config;
private ServletContext context;
// construction -------------------------------------------
protected InitialPageContext(){}
// methods ------------------------------------------------
/** Searches for the named attribute in page, request, session (if valid),
* and application scope(s) in order and returns the value associated or null.
* @see javax.servlet.jsp.PageContext#findAttribute(java.lang.String)
*/
@Override public final Object findAttribute(String name) {
Object att = null;
//page
att = this.getAttribute(name);
if (att!=null)return att;
//request
ServletRequest request=getRequest();
att = request.getAttribute(name);
if (att!=null)return att;
//session
HttpSession session=getSession();
att = session.getAttribute(name);
if (att!=null)return att;
//application
ServletContext context=getServletContext();
att = context.getAttribute(name);
if (att!=null)return att;
return null;
}
@Override public void forward(String relativeURL) throws ServletException, IOException{throw new RuntimeException();}
/** Returns the object associated with the name in the page scope
* or null if not found.
* Simple implementation: subclass may want to override.
* @see javax.servlet.jsp.PageContext#getAttribute(java.lang.String) */
@Override public Object getAttribute(String name){return page.get(name);}
/** Return the object associated with the name in the specified scope or
* null if not found.
* @see javax.servlet.jsp.PageContext#getAttribute(java.lang.String, int) */
@Override public Object getAttribute(String name, int scope){
switch (scope){
case PAGE_SCOPE:
return this.getAttribute(name);
case REQUEST_SCOPE:
ServletRequest request = this.getRequest();
return request.getAttribute(name);
case SESSION_SCOPE:
HttpSession session = this.getSession();
return session.getAttribute(name);
case APPLICATION_SCOPE:
ServletContext context = this.getServletContext();
return context.getAttribute(name);
default:
throw new IllegalArgumentException();
}
}
/** Enumerate all the attributes in a given scope.
* Simple implementation for page scope: subclass may want to override.
* The other scopes are implemented fine.
* @see javax.servlet.jsp.PageContext#getAttributeNamesInScope(int) */
@SuppressWarnings("unchecked")
@Override public Enumeration getAttributeNamesInScope(int scope) {
switch (scope){
case PAGE_SCOPE:
final Iterator keys=page.keySet().iterator();
Enumeration e = new Enumeration(){
@Override public boolean hasMoreElements(){return keys.hasNext();}
@Override public String nextElement(){return keys.next();}
};
return e;
case REQUEST_SCOPE:
ServletRequest request=getRequest();
return request.getAttributeNames();
case SESSION_SCOPE:
HttpSession session=getSession();
return session.getAttributeNames();
case APPLICATION_SCOPE:
ServletContext context=getServletContext();
return context.getAttributeNames();
default:
throw new IllegalArgumentException();
}
}
/** Get the scope where a given attribute is defined.
* @see javax.servlet.jsp.PageContext#getAttributesScope(java.lang.String) */
@Override public int getAttributesScope(String name) {
Object att = null;
//page
att = this.getAttribute(name);
if (att!=null)return PAGE_SCOPE;
//request
ServletRequest request=getRequest();
att = request.getAttribute(name);
if (att!=null)return REQUEST_SCOPE;
//session
HttpSession session=getSession();
att = session.getAttribute(name);
if (att!=null)return SESSION_SCOPE;
//application
ServletContext context=getServletContext();
att = context.getAttribute(name);
if (att!=null)return APPLICATION_SCOPE;
return 0;
}
@Override public Exception getException(){return exception;}
@Override public JspWriter getOut(){return out;}
protected void setException(Exception e){exception=e;}
protected void setOut(JspWriter o){out=o;}
protected void setRequest(HttpServletRequest r){request=r;}
protected void setResponse(HttpServletResponse r){response=r;}
protected void setSession(HttpSession s){session=s;}
@Override public HttpServlet getPage(){return servlet;}
protected void setServlet(HttpServlet s){servlet=s;}
@Override public HttpServletRequest getRequest(){return request;}
@Override public HttpServletResponse getResponse(){return response;}
@Override public ServletConfig getServletConfig(){return config;}
protected void setServletConfig(ServletConfig c){config=c;}
@Override public ServletContext getServletContext(){return context;}
protected void setServletContext(ServletContext c){context=c;}
@Override public HttpSession getSession(){return session;}
/** Delegates to handlePageException(Throwable throwable).
* @see javax.servlet.jsp.PageContext#handlePageException(java.lang.Exception) */
@Override public void handlePageException(Exception exception) throws ServletException, IOException {
Throwable throwable=exception;
handlePageException(throwable);
}
/** Not implemented.
* @see javax.servlet.jsp.PageContext#handlePageException(java.lang.Throwable) */
@Override public void handlePageException(Throwable throwable) throws ServletException, IOException{throw new RuntimeException();}
/** Not implemented.
* @see javax.servlet.jsp.PageContext#include(java.lang.String) */
@Override public void include(String arg0) throws ServletException, IOException{throw new RuntimeException();}
/** Not implemented.
* @see javax.servlet.jsp.PageContext#initialize(javax.servlet.Servlet, javax.servlet.ServletRequest, javax.servlet.ServletResponse, java.lang.String, boolean, int, boolean) */
@Override public void initialize(Servlet servlet, ServletRequest request,
ServletResponse response, String errorPageURL, boolean needsSession, int bufferSize,
boolean autoFlush) throws IOException, IllegalStateException,
IllegalArgumentException {
throw new RuntimeException();//maybe I can implement this okay.
}
/** Does nothing.
* @see javax.servlet.jsp.PageContext#release() */
@Override public void release(){}
/** Remove the object reference associated with the given name from all
* scopes. Does nothing if there is no such object.
* @see javax.servlet.jsp.PageContext#removeAttribute(java.lang.String) */
@Override public void removeAttribute(String name) {
removeAttribute(name, PAGE_SCOPE);
removeAttribute(name, REQUEST_SCOPE);
removeAttribute(name, SESSION_SCOPE);
removeAttribute(name, APPLICATION_SCOPE);
}
/** Remove the object reference associated with the specified name in the
* given scope. Does nothing if there is no such object.
* Simple implementation for page scope: subclass may want to override.
* For the other scopes, the implementation here is fine.
* @see javax.servlet.jsp.PageContext#removeAttribute(java.lang.String, int) */
@Override public void removeAttribute(String name, int scope) {
switch (scope){
case PAGE_SCOPE:
page.remove(name);
return;
case REQUEST_SCOPE:
ServletRequest request=getRequest();
request.removeAttribute(name);
return;
case SESSION_SCOPE:
HttpSession session=getSession();
session.removeAttribute(name);
return;
case APPLICATION_SCOPE:
ServletContext context=getServletContext();
context.removeAttribute(name);
return;
default:
throw new IllegalArgumentException();
}
}
/** Simple implementation: subclass may want to override.
* Register the name and value specified with page scope semantics.
* If the value passed in is null, this has the same effect as calling
* removeAttribute( name, PageContext.PAGE_SCOPE ).
* @see javax.servlet.jsp.PageContext#setAttribute(java.lang.String, java.lang.Object) */
@Override public void setAttribute(String name, Object value){page.put(name, value);}
/** Register the name and value specified with appropriate scope semantics.
* If the value passed in is null, this has the same effect as calling
* removeAttribute( name, scope ).
* @see javax.servlet.jsp.PageContext#setAttribute(java.lang.String, java.lang.Object, int) */
@Override public void setAttribute(String name, Object value, int scope) {
switch (scope){
case PAGE_SCOPE:
setAttribute(name, value);
return;
case REQUEST_SCOPE:
ServletRequest request=getRequest();
request.setAttribute(name, value);
return;
case SESSION_SCOPE:
HttpSession session=getSession();
session.setAttribute(name, value);
return;
case APPLICATION_SCOPE:
ServletContext context=getServletContext();
context.setAttribute(name, value);
return;
default:
throw new IllegalArgumentException();
}
}
@Override public void include(String arg0, boolean arg1) throws ServletException,IOException{throw new RuntimeException();}
@Override @Deprecated public ExpressionEvaluator getExpressionEvaluator(){throw new RuntimeException();}
@Override @Deprecated public VariableResolver getVariableResolver(){throw new RuntimeException();}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy