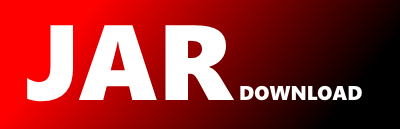
cat.inspiracio.orange.OrangeMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of orange-maven-plugin Show documentation
Show all versions of orange-maven-plugin Show documentation
Orange-maven-plugin builds template files for use with orange-servlet.
Orange Servlet provides HTML templating with server-side Java.
package cat.inspiracio.orange;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.Reader;
import java.io.Writer;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugin.logging.Log;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
import org.w3c.dom.Node;
import org.xml.sax.SAXException;
import cat.inspiracio.html.HTMLBuilder;
/** orange-maven-plugin
*
* https://maven.apache.org/guides/plugin/guide-java-plugin-development.html
*
* Call in phase generate-sources, makes .java files from the .html files. */
@Mojo(name="orange")
public class OrangeMojo extends AbstractMojo{
/** Within the maven project, the directory that contains the web app source files.
* XXX parametrise! */
private static final String WEBAPP = "src/main/webapp";
/** Within the maven project, the directory to put the generated template java sources.
* XXX parametrise! */
private static final String GENERATED_SOURCES="target/generated-sources";
// state -------------------------------------------
@Parameter(defaultValue = "${project}")
private MavenProject project;
private Log log=getLog();
/** Used for parsing file names, not really as servlet. */
private OrangeServlet orange=new OrangeServlet();
// called from mvn ---------------------------------
/** Find *.html files and generate java sources for them. */
public void execute() throws MojoExecutionException, MojoFailureException {
try{
log.info("Orange mojo");
recurse(new File(WEBAPP));
project.addCompileSourceRoot(GENERATED_SOURCES);
}
catch (Exception e) {
throw new MojoExecutionException(e.toString());
}
}
// logic ------------------------------------------
private void recurse(File f) throws Exception{
if(f.isDirectory()){
for(File n : f.listFiles())
recurse(n);
return;
}
if(isHTML(f))
generate(f);
}
/** Generate *.java from *.html
* @param f the html file
* @throws Exception */
private void generate(File f) throws Exception{
//make java class name
String path=f.getPath();
path=path.substring(WEBAPP.length());
String packageName=orange.packageName(path);
String className=orange.className(path);
//make java file name
String folder=packageName.replaceAll("\\.", "/");
String java=GENERATED_SOURCES + "/" + folder + "/" + className + ".java";
new File(GENERATED_SOURCES + "/" + folder).mkdirs();
Node n=null;
try(Reader r=new FileReader(f)){
n=parse(r);
}
try(Writer w=new FileWriter(new File(java))){
Programmer p=new Programmer(w);
p.setLog(log);
p.setPackage(packageName);
p.setClass(className);
p.generate(n);
}
}
/** Parses real html5.
* @see cat.inspiracio.html.HTMLServlet#parse(java.io.Reader) */
private Node parse(Reader reader) throws IOException, SAXException{
HTMLBuilder builder=new HTMLBuilder();
return builder.parse(reader);
}
private boolean isHTML(File f){
String name=f.getName();
return name.endsWith(".html");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy