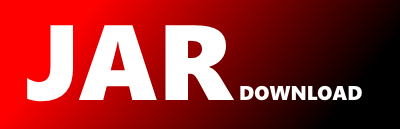
cat.inspiracio.orange.Template Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of orange-servlet Show documentation
Show all versions of orange-servlet Show documentation
Orange Servlet provides HTML templating with server-side Java.
OrangeServlet is a servlet that is registered on *.html.
It reads the html file at the right location according to URL.
It renders the file as html, executing some scripting:
* data-if="E" attributes for server-side conditional,
* data-for="T x : E" attributes for server-side loops,
* data-import="C" attributes for server-side class imports,
* data-substitute="file.html" substitute an element with the processed contents of a file,
* ${expressions} in element bodies and attribute values.
package cat.inspiracio.orange;
import java.io.IOException;
import javax.servlet.ServletConfig;
import javax.servlet.ServletContext;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import javax.servlet.jsp.JspWriter;
import javax.servlet.jsp.PageContext;
public abstract class Template {
private static final String NL="\n";
// state -----------------------------------------------
//All these variables are visible in the write() method.
protected JspWriter out;
protected HttpServletRequest request;
protected HttpServletResponse response;
protected HttpSession session;
protected PageContext pageContext;
protected ServletContext application;
protected ServletConfig config;
protected Template page;
protected Throwable exception;
// construction --------------------------------
public Template(){}
/** The only setter you need to call.
* It sets all fields. */
public final void setPageContext(PageContext pc){
out=pc.getOut();
pageContext=pc;
request=(HttpServletRequest)pc.getRequest();
response=(HttpServletResponse)pc.getResponse();
session=pc.getSession();
application=pc.getServletContext();
config=pc.getServletConfig();
page=this;//Or better?
exception=pc.getException();
}
// abstract method -----------------------------
/** This is the method that template must implement.
* @throws Exception Java-islands threw an exception. */
public abstract void write()throws Exception;
// orange logic ------------------------------
/** Called for an element with attribute data-substitute=v.
*
* The attribute value is a path. The servlet reads a file at the path.
* The file contains an HTML fragment. The fragment is inserted in the
* document, substituting the original element.
*
* Example:
*
*
* The attribute value is literally a path. It is not a javascript expression
* which when evaluated results in a path.
*
* The path is relative to the location of the document in which it occurs,
* or absolute if it starts with /.
*
* @throws IOException */
protected final void substitute(String v) throws Exception{
String n=resolve(v);
//instantiate the class and call it
@SuppressWarnings("unchecked")
Classc=(Class)Class.forName(n);
Template t=c.newInstance();
t.setPageContext(pageContext);
t.write();
}
/** Gets the class name of this template.
*
* Package-visible so that tests can fake it. */
String getClassName(){return getClass().getName();}
/** Resolves path v relative to the path of this template.
*
* Package-visible for tests.
*
* @param v relative or absolute path, like "hr.html" or "/cacti/cactus.html".
* Absolute path must not contain "." or ".." directories.
*
* @return fully qualified class name of v */
final String resolve(String v){
//XXX Unite with OrangeServlet.packageName(path) and className(path).
String n="cat.inspiracio.orange.webapp.hr";//not the real class name yet
//absolute path, May it contain ".." or "."?
if(v.startsWith("/")){
//absolute, not relative to current template
v=v.substring(1);//drop initial "/"
v=v.substring(0, v.length()-5);//drop final ".html"
v=v.replace('-', '_');
v=v.replace('/', '.');//not correct if there is ".." or "."
n="cat.inspiracio.orange.webapp." + v;
return n;
}
//resolve v with respect to the current template
else{
//Improve this
String current=getClassName();
//treat ".." and "."
String[]parts=current.split("\\.");
v=v.substring(0, v.length()-5);//drop final ".html"
v=v.replace('-', '_');
String[]vs=v.split("/");//first split
String[] cs=new String[parts.length-1 + vs.length];
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy