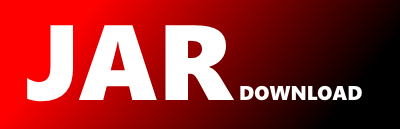
cat.inspiracio.orange.OrangeServlet Maven / Gradle / Ivy
/* Copyright 2019 Alexander Bunkenburg
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package cat.inspiracio.orange;
import java.io.IOException;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.jsp.PageContext;
import cat.inspiracio.servlet.jsp.ServletPageContext;
import cat.inspiracio.util.Timber;
/** For requests to orange templates. */
//@WebServlet("*.html")
public class OrangeServlet extends HttpServlet{
// not state --------------
private static final long serialVersionUID = -1871765722924200945L;
private Resolver resolver=new Resolver();
private WebXml webXml=new WebXml(this);
// public methods ----------
@Override protected void doGet(HttpServletRequest request, HttpServletResponse response)throws ServletException, IOException {
setContentType(response);
try{
Template t = null;
try {
t = template(request, response);//ClassNotFoundException
}
//Can not find a template class: 404
//OrangeServlet translates not finding the template class to 404.
catch(ClassNotFoundException e){
error(request, 404, e);
response.sendError(404);
return;
}
t.render();//Exception, may be anything from the Java-islands
timber(response);
}
catch(Exception e){
error(request, 500, e);
response.sendError(500);
}
}
/** For POST, do the same as for GET.
* The html can discriminate and react. */
@Override protected void doPost(HttpServletRequest request, HttpServletResponse response)throws ServletException, IOException {
doGet(request, response);
}
// helpers ---------------------------------------------------------
private void setContentType(HttpServletResponse response){
//make this depend on file extension?
response.setContentType("text/html; charset=utf-8");
}
/** Find a template class and instantiate it.
* @param request
* @param response
* @throws ClassNotFoundException if the template can't be found. That's 404.
* @throws NoSuchMethodException Template class lacks constructor. Orange maven plugin is broken.
* @throws InstantiationException Template instantiation failed. Orange maven plugin is broken.
* @throws IllegalAccessException Template instantiation failed. Orange maven plugin is broken.
* @throws InvocationTargetException Template instantiation failed. Orange maven plugin is broken.
* */
Template template(HttpServletRequest request, HttpServletResponse response) throws ClassNotFoundException, NoSuchMethodException, InstantiationException, IllegalAccessException, InvocationTargetException {
//Is it necessary to get reflection out of request time?
String fqcn = fully(request);
ServletPageContext pc = new ServletPageContext(this, request, response);
Class extends Template>c = Class.forName(fqcn).asSubclass(Template.class);//ClassNotFoundException
Constructor extends Template> cons = c.getConstructor(PageContext.class);
Template t = cons.newInstance(pc);
return t;
}
/** An exception has occurred. Puts some attributes into the request.
* Spec https://javaee.github.io/servlet-spec/downloads/servlet-3.1/Final/servlet-3_1-final.pdf
* */
private void error(HttpServletRequest request, int status, Throwable e){
request.setAttribute("javax.servlet.error.status_code", status);
request.setAttribute("javax.servlet.error.exception_type", e.getClass());
request.setAttribute("javax.servlet.error.message", e.getMessage());
request.setAttribute("javax.servlet.error.exception", e);
request.setAttribute("javax.servlet.error.request_uri", request.getRequestURI());
request.setAttribute("javax.servlet.error.servlet_name", getServletName());
}
/** Makes full java class name from request path.
* Example: GET /index.html --> cat.inspiracio.orange.index,java */
private String fully(HttpServletRequest request){
String contextPath = request.getContextPath();
String uri = request.getRequestURI();
if(contextPath!=null && uri.startsWith(contextPath))
uri = uri.substring(contextPath.length());
// Tomcat has directed to welcome file, even though request.getRequestURI() == "/".
if("/".equals(uri)) {
uri = request.getServletPath();// "/index.html"
//System.out.println(request.getAttribute(RequestDispatcher.FORWARD_REQUEST_URI));//null
//System.out.println(request.getRequestURL());//https://localhost:8443/web/
//System.out.println(request.getPathInfo());//null
//uri = webXml.getWelcomeFile();//works, but quite hacky
}
return resolver.fqcn(uri);
}
/** Maybe write timber output to page.
* Only if it's .html and there is something to write.
* @throws IOException */
private void timber(HttpServletResponse response) throws IOException{
String type=response.getContentType(); //text/html;charset=utf-8
boolean isHTML= type!=null && type.startsWith("text/html");
if(isHTML) {
String timber=Timber.string();
if(0");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy