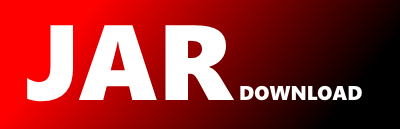
cc.kave.repackaged.jayes.internal.util.AddressCalc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cc.kave.repackaged.jayes Show documentation
Show all versions of cc.kave.repackaged.jayes Show documentation
Repackaging of Jayes (Eclipse Code Recommenders) to make it available in Maven.
The newest version!
/**
* Copyright (c) 2011 Michael Kutschke.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* Michael Kutschke - initial API and implementation.
*/
package cc.kave.repackaged.jayes.internal.util;
import java.util.HashMap;
import java.util.Map;
import cc.kave.repackaged.jayes.factor.AbstractFactor;
import cc.kave.repackaged.jayes.util.MathUtils;
public final class AddressCalc {
private AddressCalc() {
}
public static void incrementMultiDimensionalCounter(final int[] counter, final int[] dimensions) {
int dimension = counter.length - 1;
counter[dimension]++;
while (counter[dimension] == dimensions[dimension]) {
// overflow, assume the counter was valid before and less than the
// maximal counter
counter[dimension] = 0;
dimension--;
counter[dimension]++;
}
}
/**
* computes a mapping from the factors' addresses to the corresponding index of the flat value array of a factor
* consisting of the dimensions with the given dimensionIDs. dimensionIDs is expected to be a superset of the
* dimension ids of the factor
* @param factor the factor
* @param dimensionIDs array of dimension ids
* @return mapping
*/
public static int[] computeLinearMap(AbstractFactor factor, int... dimensionIDs) {
return computeLinearMap(computeIdToDimensionIndexMap(factor), factor.getDimensions(),
dimensionIDs);
}
private static int[] computeLinearMap(Map foreignIdToIndex, int[] foreignDimensions,
int[] dimensionIds) {
int[] kernel = new int[dimensionIds.length];
for (int i = 0; i < kernel.length; i++) {
int dimensionId = dimensionIds[i];
if (foreignIdToIndex.containsKey(dimensionId)) {
kernel[i] = MathUtils.productOfRange(foreignDimensions, foreignIdToIndex.get(dimensionId) + 1,
foreignDimensions.length);
}
}
return kernel;
}
private static Map computeIdToDimensionIndexMap(AbstractFactor factor) {
Map foreignIds = new HashMap();
for (int i = 0; i < factor.getDimensionIDs().length; i++) {
foreignIds.put(factor.getDimensionIDs()[i], i);
}
return foreignIds;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy