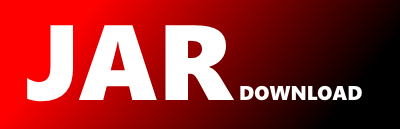
cc.neckbeard.utils.UuidConverter Maven / Gradle / Ivy
package cc.neckbeard.utils;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.Collection;
import java.util.HashSet;
import java.util.Set;
import java.util.UUID;
import java.util.regex.Pattern;
public class UuidConverter {
private static final String FORMAT = "$1-$2-$3-$4-$5";
private static final Pattern PATTERN = Pattern.compile("([0-9a-fA-F]{8})-?([0-9a-fA-F]{4})-?([0-9a-fA-F]{4})-?([0-9a-fA-F]{4})-?([0-9a-fA-F]{12})");
/**
* Generates a UUID Object from a UUID String.
* The String can either contain a dashed or undashed UUID.
*
* @param string UUID String
* @return UUID Object
* @throws IllegalArgumentException Thrown if UUID is not valid.
*/
public static @Nullable UUID of(@NotNull final String string) {
if (!PATTERN.matcher(string).matches()) return null;
try {
return UUID.fromString(string.replaceFirst(PATTERN.toString(), FORMAT));
} catch (IllegalArgumentException ignored) {
}
return null;
}
/**
* Converts a Collection of UUID objects to a Set of Strings.
*
* @return String representations of UUIDs stored in a Set.
*/
public static @NotNull Set stringify(@NotNull final Set uuids) {
Set set = new HashSet<>();
for (UUID uuid : uuids) {
if (uuid == null) continue;
set.add(uuid.toString());
}
return set;
}
/**
* Converts a Collection of UUID objects to a Set of Strings.
*
* @return String representations of UUIDs stored in a Set.
*/
public static @NotNull Set stringify(@NotNull final Collection uuids) {
return stringify(uuids);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy