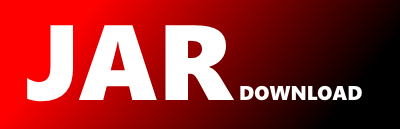
cc.owoo.godpen.thread.CacheThreadPool Maven / Gradle / Ivy
package cc.owoo.godpen.thread;
import java.util.LinkedList;
/**
* 缓存线程池
* Created by nimensei
* 2022-04-06 下午 05:48
**/
public class CacheThreadPool {
private final Object lock = new Object();//线程锁
private int count;// 最大线程数
private int now;//当前线程数
private final LinkedList list = new LinkedList<>();// 等待运行的线程
public CacheThreadPool(int count) {
setMaxThreadCount(count);
}
/**
* 设置最大线程数
*
* @param count 最大线程数
*/
public void setMaxThreadCount(int count) {
if (count <= 0)
throw new IllegalArgumentException("线程数不能小于1:" + count);
this.count = count;
}
/**
* 获取最大线程数
*
* @return 最大线程数
*/
public int getMaxThreadCount() {
return count;
}
/**
* 获取当前线程池数量
*
* @return 当前线程池数量
*/
public int getNowThreadCount() {
return now;
}
/**
* 执行
*
* @param runnable 回调函数
*/
public void execute(Runnable runnable) {
if (runnable == null)
throw new NullPointerException("线程运行回调函数不能为空");
synchronized (lock) {
list.add(runnable);
}
execute();
}
/**
* 执行线程池
*/
private void execute() {
Runnable runnable;
synchronized (lock) {
if (list.size() == 0 || now >= count)
return;
runnable = list.removeFirst();
++now;
}
Threads.run(() -> executeTask(runnable));
}
/**
* 执行任务
*
* @param runnable 任务回调函数
*/
private void executeTask(Runnable runnable) {
runnable.run();
synchronized (lock) {
--now;
}
execute();
}
/**
* 线程全部结束
*/
public void finish() {
while (now != 0)
Threads.delay(10);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy