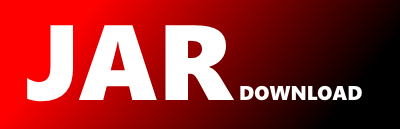
cc.protea.foundation.providers.DateParamConverterProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of foundation Show documentation
Show all versions of foundation Show documentation
Integrate compatible versions of many external systems to create a REST/HTTP server foundation
package cc.protea.foundation.providers;
import org.apache.commons.lang3.StringUtils;
import javax.ws.rs.ext.ParamConverter;
import javax.ws.rs.ext.ParamConverterProvider;
import javax.ws.rs.ext.Provider;
import java.lang.annotation.Annotation;
import java.lang.reflect.Type;
import java.text.SimpleDateFormat;
import java.util.Date;
@Provider
public class DateParamConverterProvider implements ParamConverterProvider {
@SuppressWarnings({ "rawtypes", "unchecked" })
@Override
public ParamConverter getConverter(Class type, Type genericType, Annotation[] annotations) {
if (type.equals(Date.class)) {
return new DateParamConverter();
}
return null;
}
static Date dateToString(String in) {
try {
in = StringUtils.trimToEmpty(in);
switch (in.length()) {
case 8:
return new Date(new SimpleDateFormat("yyyyMMdd").parse(in).getTime());
case 10:
return new Date(new SimpleDateFormat("yyyy-MM-dd").parse(in).getTime());
case 19:
if (in.contains("T")) {
return new Date(new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss").parse(in).getTime());
} else {
return new Date(new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").parse(in).getTime());
}
case 24:
if (StringUtils.endsWith(in, "Z")) {
in = StringUtils.substring(in, 0, 19) + "Z";
}
// fall through
case 20:
return new Date(new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss'Z'").parse(in).getTime());
}
if (StringUtils.isNumeric(in)) {
return new Date(Long.parseLong(in));
}
} catch (Exception e) {
throw new IllegalArgumentException("Could not convert to a date: '" + in + "'", e);
}
throw new IllegalArgumentException("Could not convert to a date: '" + in + "'");
}
private static class DateParamConverter implements ParamConverter {
@Override
public Date fromString(String in) {
return dateToString(in);
}
@Override
public String toString(Date value) {
return value.toString();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy