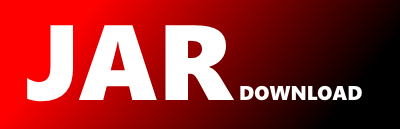
cc.protea.foundation.util.KeyUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of foundation Show documentation
Show all versions of foundation Show documentation
Integrate compatible versions of many external systems to create a REST/HTTP server foundation
package cc.protea.foundation.util;
import org.apache.commons.lang3.CharUtils;
import org.apache.commons.lang3.StringUtils;
public class KeyUtil {
public static String toString(final Number key) {
if (key == null || key.longValue() < 1 || key.longValue() > 999999999999L) {
return null;
}
boolean big = key.longValue() > 999999999;
int numLength = big ? 12 : 9;
String keyString = StringUtils.leftPad(String.valueOf(key), numLength, '0');
long interesting;
try {
interesting = Long.parseLong(StringUtils.reverse(keyString));
} catch (NumberFormatException e) {
return null;
}
String base34 = KeyUtil.longToBase34(interesting, big);
return base34;
}
public static String toString(final Object o) {
if (o == null || ! Number.class.isAssignableFrom(o.getClass())) {
return null;
}
return KeyUtil.toString((Number)o);
}
public static Long toKey(final String candidate) {
String base34 = KeyUtil.sanitizeKey(candidate);
if (base34 == null) {
return null;
}
if (base34.length() != 6 && base34.length() != 8) {
return null;
}
int numLength = base34.length() == 6 ? 9 : 12;
long interesting = KeyUtil.base34ToLong(base34);
String reversedString = StringUtils.leftPad(String.valueOf(interesting), numLength, '0');
String keyString = StringUtils.reverse(reversedString);
return Long.valueOf(keyString, 10);
}
public static Long toKey(final Object o) {
return o == null ? null : KeyUtil.toKey(o.toString());
}
public static boolean isKey(final String candidate) {
return KeyUtil.toKey(candidate) != null;
}
public static boolean isKey(final Object o) {
return o == null ? false : KeyUtil.isKey(o.toString());
}
/**
* Expects a long that is equal or less than 26^8 (1,785,793,904,896) and returns a modified base 34 representation (should be 8 bytes) This will never include a 0 or a 1
*/
static String longToBase34(final long in, final boolean big) {
int finishedLength = big ? 8 : 6;
String base34 = Long.toString(in, 34);
int len = base34.length();
StringBuilder accum = new StringBuilder();
int ycount = Math.max(finishedLength - len, 0);
for (int i = 0; i < ycount; i++) {
accum.append('Y');
}
for (int i = 0; i < len; i++) {
char c = base34.charAt(i);
if (c == '0') {
accum.append('Y');
} else if (c == '1') {
accum.append('Z');
} else {
accum.append(Character.toUpperCase(c));
}
}
return accum.toString();
}
/**
* Expects an eight byte modified base 34 representation and returns a long that is equal or less than 34^8 (1,785,793,904,896)
*/
static Long base34ToLong(final String string) {
if (string == null) {
return null;
}
StringBuilder sb = new StringBuilder();
for (int i = 0; i < string.length(); ++i) {
char c = string.charAt(i);
switch (c) {
case '0':
sb.append('O');
break;
case '1':
sb.append('I');
break;
case 'Y':
case 'y':
sb.append('0');
break;
case 'Z':
case 'z':
sb.append('1');
break;
default:
sb.append(c);
}
}
String base34 = sb.toString();
try {
return Long.parseLong(base34, 34);
} catch (NumberFormatException e) {
return null;
}
}
static String sanitizeKey(final String candidate) {
if (candidate == null) {
return null;
}
StringBuilder sb = new StringBuilder();
for (int i = 0; i < candidate.length(); i++) {
char ch = candidate.charAt(i);
if (CharUtils.isAsciiAlphanumeric(ch)) {
sb.append(ch);
}
}
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy